Advanced CSS Grid Techniques: Designing Complex Web Page Layouts
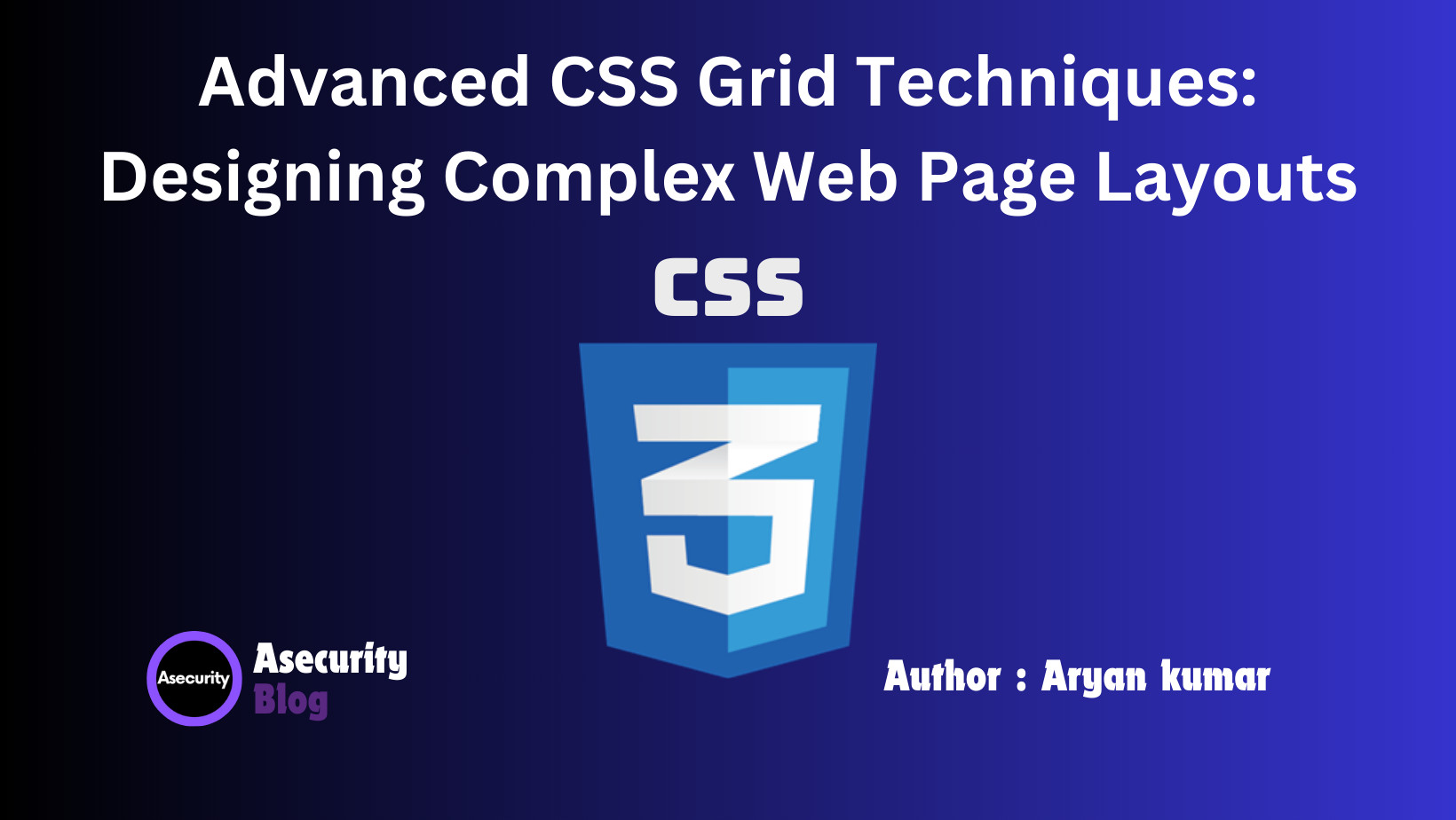
As web design evolves, the need for more complex, versatile, and responsive layouts becomes increasingly essential. CSS Grid Layout, often simply referred to as CSS Grid, is a powerful two-dimensional layout system designed specifically to handle these requirements. Unlike Flexbox, which is one-dimensional and deals primarily with either rows or columns, CSS Grid allows you to control both at the same time, providing a comprehensive system for creating intricate layouts.
Understanding the CSS Grid
CSS Grid introduces a new way of thinking about layout, moving away from traditional methods that rely on floats and positioning. It enables the creation of grid-based layouts that are both robust and responsive, perfect for any modern website.
Key Concepts:
- Grid Container and Grid Items:
- Grid Container: The parent element that defines the grid layout. It is created by setting
display: grid;
ordisplay: inline-grid;
on an element. - Grid Items: The children of the grid container, which are automatically placed into the grid cells.
- Grid Container: The parent element that defines the grid layout. It is created by setting
- Grid Lines, Tracks, and Cells:
- Grid Lines: The lines that divide the grid into rows and columns. These are numbered starting from 1 at the edge of the grid.
- Grid Tracks: The space between two adjacent grid lines. This can be a row track (horizontal) or a column track (vertical).
- Grid Cells: The space between four intersecting grid lines. These are the individual units of the grid where items are placed.
- Grid Areas:
- Grid Areas: Rectangular sections within the grid layout that can span multiple rows and columns. You can define grid areas to group items together, making them easier to manage and style.
Setting Up a Basic Grid
To get started with CSS Grid, let’s create a simple layout that consists of a header, main content area, and footer:
<div class="grid-container">
<header class="header">Header</header>
<main class="main">Main Content</main>
<footer class="footer">Footer</footer>
</div>
Here’s the CSS to define a basic grid layout:
.grid-container {
display: grid;
grid-template-rows: 100px 1fr 50px;
grid-template-columns: 1fr;
grid-template-areas:
"header"
"main"
"footer";
height: 100vh;
}
.header {
grid-area: header;
background-color: #333;
color: white;
text-align: center;
}
.main {
grid-area: main;
background-color: #f4f4f4;
}
.footer {
grid-area: footer;
background-color: #333;
color: white;
text-align: center;
}
Explanation:
display: grid;
: Turns the.grid-container
into a grid container.grid-template-rows
andgrid-template-columns
: Define the structure of the grid by specifying the size of rows and columns.grid-template-areas
: Names different areas of the grid, allowing you to easily place items within those areas.- The
.header
,.main
, and.footer
classes assign grid areas to each grid item, based on the definedgrid-template-areas
.
Advanced Grid Techniques
Now that you’ve mastered the basics, let’s explore some advanced techniques that CSS Grid offers:
- Explicit vs. Implicit Grids:
- Explicit Grids: Defined using
grid-template-rows
andgrid-template-columns
. These specify exactly where grid lines will appear. - Implicit Grids: Created when grid items are placed outside the explicitly defined grid. The browser automatically creates extra rows or columns to accommodate these items.
- Explicit Grids: Defined using
- Grid Auto Placement:
- Grid Auto Rows and Auto Columns: You can define
grid-auto-rows
andgrid-auto-columns
to automatically size new rows and columns added to the grid. grid-auto-flow
: This property controls how the auto-placement algorithm works, with options likerow
,column
,dense
, etc., to control the placement of items.
- Grid Auto Rows and Auto Columns: You can define
- Fractional Units (fr):
fr
unit: A flexible length that allows you to allocate available space within the grid container. For instance, using1fr 2fr
divides the space so that the first column gets 1/3 of the space and the second gets 2/3.
- Aligning Items with CSS Grid:
align-items
andjustify-items
: Control the alignment of grid items within their grid cells.align-content
andjustify-content
: Control the alignment of the grid tracks (rows or columns) within the grid container.
Creating a Complex Layout with CSS Grid
To illustrate the power of CSS Grid, let's build a more complex layout:
<div class="grid-container">
<header class="header">Header</header>
<nav class="nav">Navigation</nav>
<aside class="sidebar">Sidebar</aside>
<main class="main">Main Content</main>
<footer class="footer">Footer</footer>
</div>
And the corresponding CSS:
.grid-container {
display: grid;
grid-template-areas:
"header header header"
"nav main sidebar"
"footer footer footer";
grid-template-columns: 1fr 3fr 1fr;
grid-template-rows: 100px 1fr 50px;
gap: 10px;
height: 100vh;
}
.header {
grid-area: header;
background-color: #333;
color: white;
text-align: center;
}
.nav {
grid-area: nav;
background-color: #444;
color: white;
}
.sidebar {
grid-area: sidebar;
background-color: #555;
color: white;
}
.main {
grid-area: main;
background-color: #f4f4f4;
}
.footer {
grid-area: footer;
background-color: #333;
color: white;
text-align: center;
}
Explanation:
- Grid Layout: The
grid-template-areas
property is used to define a grid layout that spans three rows and three columns, with different areas assigned for each part of the layout. - Responsive Design: The layout is responsive and adjusts based on the available screen space, with the main content taking up most of the space (
3fr
for the main column).
Conclusion
CSS Grid is an incredibly powerful tool for web designers and developers, enabling the creation of complex, responsive layouts with minimal effort. By mastering the grid system, you can design web pages that are not only visually appealing but also highly adaptable to different devices and screen sizes.
With CSS Grid, the possibilities are virtually limitless. Experiment with various grid properties, combine them creatively, and watch as your web designs become more structured, responsive, and visually compelling.
In the next installment of this series, we’ll explore Responsive Design Principles and how to craft mobile-friendly websites using a combination of CSS techniques. Stay tuned!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript