Control Structures in JavaScript: Mastering Conditionals and Loops
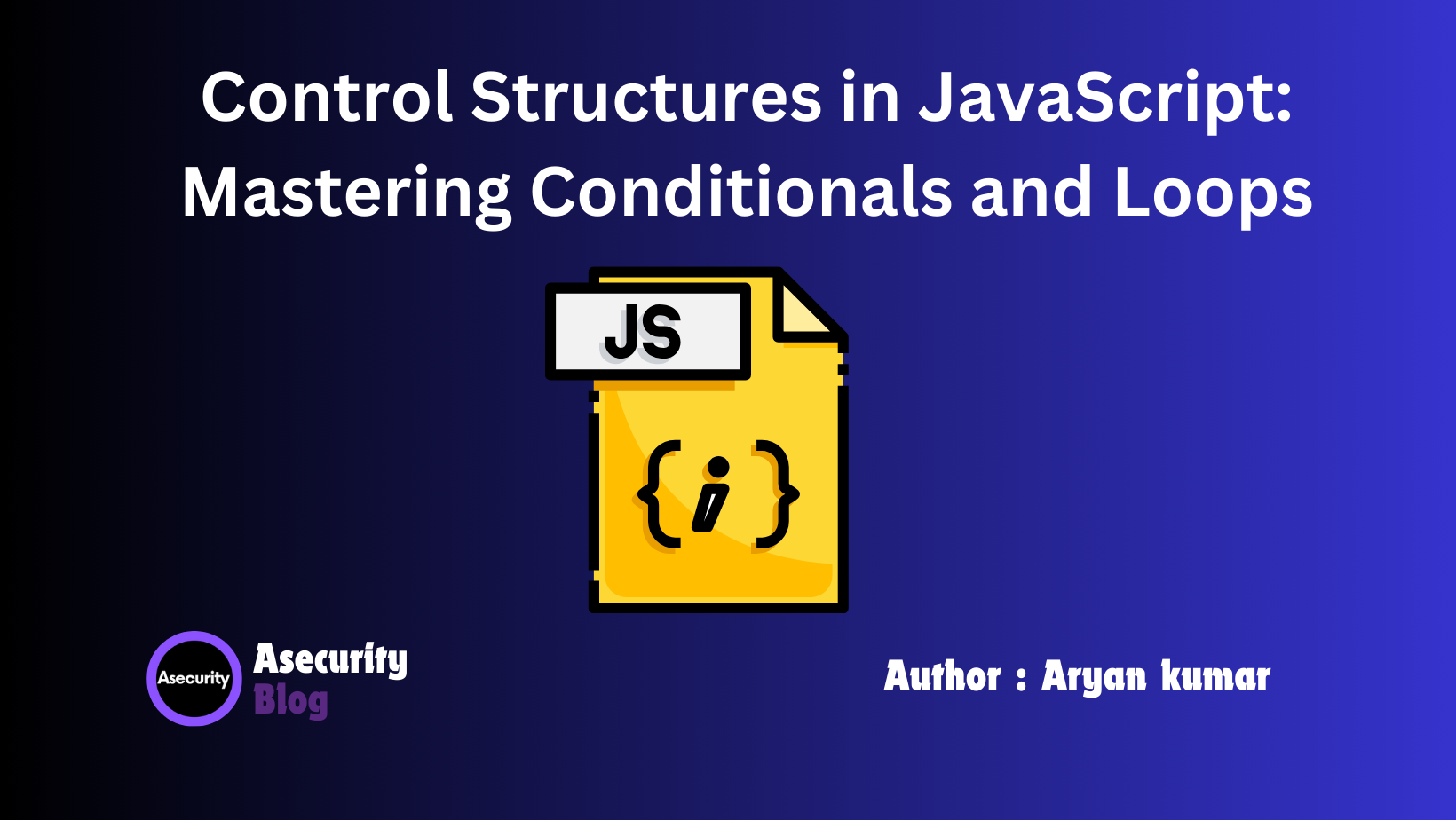
In your journey of mastering JavaScript, understanding control structures is key. Control structures allow you to dictate the flow of your program, enabling it to make decisions, perform repetitive tasks, and respond to user inputs. In this blog, we’ll explore the two main types of control structures: conditionals and loops, and how to use them to create more dynamic and interactive web pages.
Understanding Conditionals: Making Decisions in Code
Conditionals allow your JavaScript code to make decisions based on certain conditions. The most common type of conditional is the if
statement, which runs a block of code if a specified condition is true.
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote.");
}
In this example, the condition checks whether the variable age
is greater than or equal to 18. If true, the message "You are eligible to vote" is logged to the console.
else
and else if
Statements
Sometimes, you need to handle multiple scenarios. This is where else
and else if
come into play.
let score = 75;
if (score >= 90) {
console.log("Excellent!");
} else if (score >= 50) {
console.log("Good job!");
} else {
console.log("Keep trying!");
}
In this example, the code checks the value of score
and responds with different messages depending on the range the score falls into.
Ternary Operator: A Shortcut for Conditionals
The ternary operator is a shorter way of writing if-else
statements. It is especially useful for simple conditionals.
let isMember = true;
let discount = isMember ? 10 : 0;
console.log(discount);
Here, if isMember
is true
, the variable discount
will be assigned 10
; otherwise, it will be 0
.
Loops: Repeating Tasks Efficiently
Loops allow you to run a block of code multiple times. This is incredibly useful for tasks that require repetition, like processing items in an array or performing a task until a condition is met.
for
Loop: A Classic Loop
The for
loop is one of the most commonly used loops in JavaScript. It repeats a block of code a specified number of times.
for (let i = 0; i < 5; i++) {
console.log(`This is loop iteration number ${i}`);
}
In this example, the loop runs five times, logging a message to the console during each iteration.
while
Loop: Repeating Based on a Condition
The while
loop repeats a block of code as long as a specified condition is true.
let count = 0;
while (count < 3) {
console.log(`Count is ${count}`);
count++;
}
In this case, the loop continues running as long as the value of count
is less than 3.
do...while
Loop: Ensuring Code Runs at Least Once
The do...while
loop is similar to the while
loop, but it guarantees that the code block runs at least once, even if the condition is false initially.
let num = 5;
do {
console.log(`Number is ${num}`);
num--;
} while (num > 0);
Here, the code inside the do
block will run first before checking if the condition is true.
break
and continue
: Controlling Loop Flow
Sometimes, you may need to stop a loop before it finishes or skip an iteration. This is where break
and continue
come in handy.
break
: Exiting a Loop Early
for (let i = 0; i < 10; i++) {
if (i === 5) {
break;
}
console.log(i);
}
In this case, the loop stops once i
reaches 5.
continue
: Skipping to the Next Iteration
for (let i = 0; i < 10; i++) {
if (i === 5) {
continue;
}
console.log(i);
}
This loop skips the iteration where i
is equal to 5, but continues running for other values.
Conclusion
Mastering control structures like conditionals and loops is essential for creating more dynamic and functional JavaScript programs. Conditionals allow your code to make decisions, while loops enable you to perform repetitive tasks efficiently. With these tools, you can write more powerful, flexible, and interactive scripts that respond to user inputs and streamline repetitive processes. As you continue developing your JavaScript skills, these control structures will become foundational to solving problems and implementing advanced logic in your code.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript