Data Handling with JavaScript: Mastering Arrays and Objects
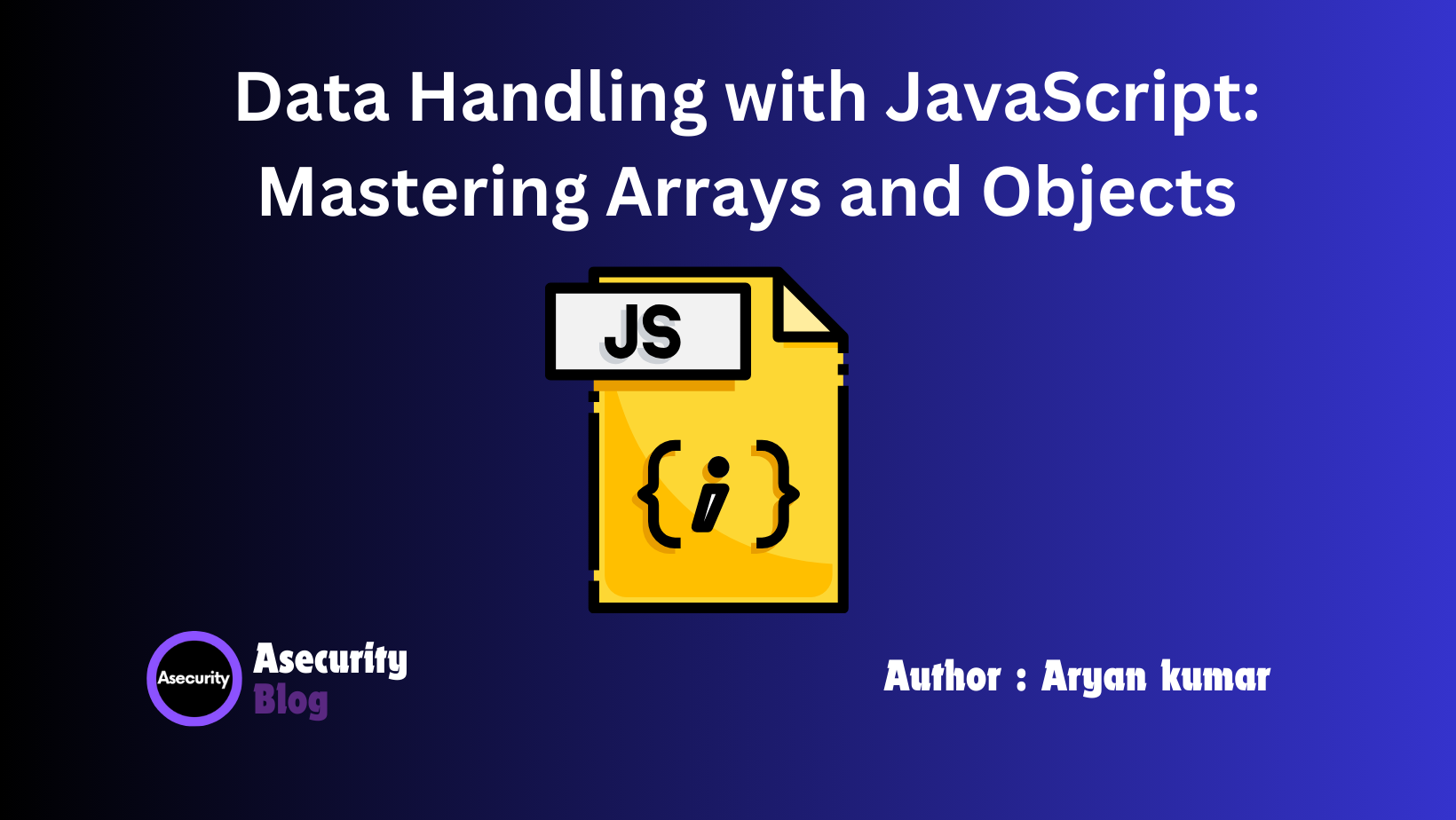
Introduction
Handling data efficiently is crucial for web development, and JavaScript provides powerful tools to manage data using arrays and objects. These data structures allow you to store, manipulate, and organize data in ways that bring your web applications to life. In this blog, we’ll dive deep into mastering arrays and objects in JavaScript, equipping you with the knowledge needed to handle data with confidence.
1. Understanding JavaScript Arrays
What is an Array?
An array is a data structure used to store multiple values in a single variable. Arrays are useful for storing ordered collections of items, such as lists of numbers, strings, or even other arrays. They are one of the most commonly used data structures in JavaScript, offering powerful methods for manipulating collections of data.
Creating Arrays
There are multiple ways to create arrays in JavaScript:
- Array Literals:
let fruits = ['apple', 'banana', 'cherry'];
- Using the
new Array()
Constructor:
let numbers = new Array(1, 2, 3, 4, 5);
Accessing Array Elements
Elements in an array are accessed using their index, starting from 0 for the first element.
console.log(fruits[0]); // Outputs: apple
fruits[1] = 'mango'; // Changing the second element to 'mango'
Common Array Methods
- Adding and Removing Elements:
.push()
: Adds an element to the end..pop()
: Removes the last element..shift()
: Removes the first element..unshift()
: Adds an element to the beginning.
fruits.push('orange'); // Adds 'orange' to the end of the array
fruits.pop(); // Removes the last element ('orange')
Iteration Methods:
forEach()
: Executes a function for each element.map()
: Creates a new array with the results of calling a function on every element.filter()
: Creates a new array with all elements that pass a test.
fruits.forEach(fruit => console.log(fruit)); // Logs each fruit
let filteredFruits = fruits.filter(fruit => fruit !== 'mango');
- Searching and Sorting:
find()
: Returns the first element that matches a condition.sort()
: Sorts the elements of the array.
2. Understanding JavaScript Objects
What is an Object?
Objects in JavaScript are collections of key-value pairs. They can represent complex entities like a person, a car, or any other structured data. Unlike arrays, which store data in an ordered sequence, objects store data in a structured way, making them ideal for storing related information.
Creating Objects
- Object Literals:
let person = {
name: 'John',
age: 30,
job: 'Developer'
};
- Using the
new Object()
Constructor:
let car = new Object();
car.make = 'Toyota';
car.model = 'Corolla';
Accessing and Modifying Object Properties
- Dot Notation:
console.log(person.name); // Outputs: John
person.age = 31; // Updates the age to 31
Bracket Notation:
console.log(person['job']); // Outputs: Developer
Common Object Methods
Object.keys()
: Returns an array of a given object's own enumerable property names.Object.values()
: Returns an array of a given object's own enumerable property values.Object.entries()
: Returns an array of a given object's own enumerable string-keyed property [key, value] pairs.
3. Arrays vs. Objects: When to Use Which?
- Arrays are ideal when the data is ordered and needs indexing, like lists, queues, or stacks.
- Objects are perfect when dealing with complex data where items are named and not ordered, such as user profiles or settings.
4. Combining Arrays and Objects
Arrays and objects can be combined to create complex data structures. For example, you can have an array of objects representing a list of users:
let users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 }
];
5. Practical Example: Building a Simple Contact List
Let’s create a simple contact list using arrays and objects.
let contacts = [
{ name: 'John Doe', phone: '123-456-7890' },
{ name: 'Jane Smith', phone: '098-765-4321' }
];
// Adding a new contact
contacts.push({ name: 'Sam Wilson', phone: '555-555-5555' });
// Updating a contact
contacts[0].phone = '111-222-3333';
// Removing a contact
contacts.splice(1, 1); // Removes the second contact
Conclusion
Mastering arrays and objects is essential for any JavaScript developer. They are the backbone of data manipulation and are frequently used in web applications, making them crucial to learn and understand. Practice using arrays and objects in your projects to enhance your skills and become proficient in managing data with JavaScript.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript