Debugging and Error Handling in JavaScript: Techniques and Tools
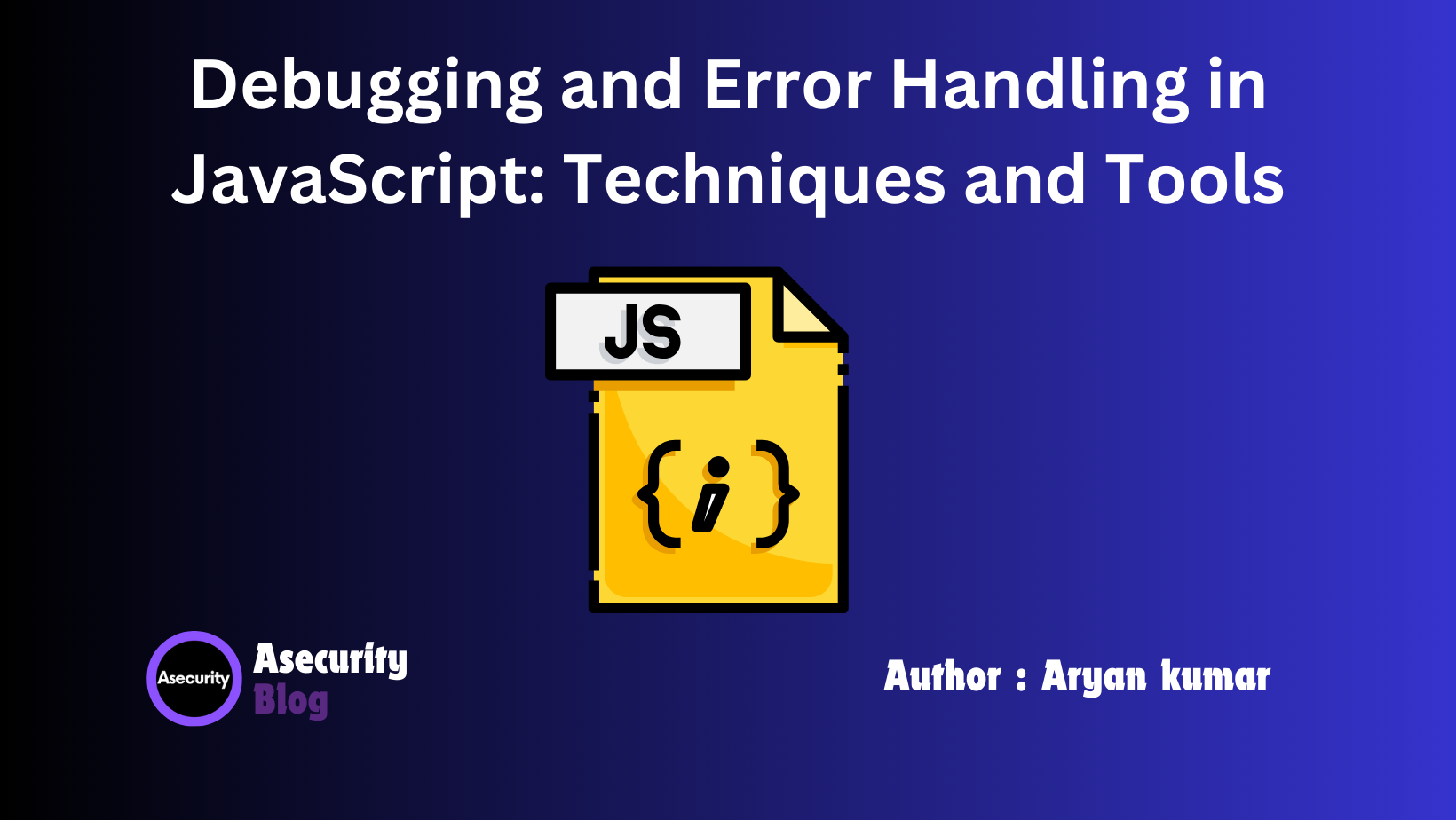
When working with JavaScript, debugging and error handling are crucial skills that can save you countless hours of frustration. Bugs are inevitable when writing code, but with the right techniques and tools, you can quickly identify and fix errors, ensuring that your web applications run smoothly. In this blog, we'll explore some of the best practices for debugging JavaScript code and handling errors gracefully.
1. Understanding JavaScript Errors
JavaScript errors generally fall into three categories:
- Syntax Errors: These occur when there's a mistake in the structure of your code, such as missing parentheses or brackets.
- Runtime Errors: These happen when the script tries to perform an operation that it can't complete, like accessing a non-existent variable.
- Logical Errors: These occur when your code runs, but it doesn’t behave as expected due to incorrect logic.
Each type of error requires a different approach to fix, but the common goal is to ensure that your code runs error-free or handles errors gracefully.
2. Using console.log()
for Debugging
One of the most basic and effective debugging techniques is using the console.log()
method. It allows you to output variable values, check the flow of code execution, and quickly spot where things go wrong.
For example:
let total = calculateTotal(price, quantity);
console.log('Total is:', total);
This simple method can help you trace the source of an issue by logging different parts of your code and variables.
3. Leveraging the Browser's Developer Tools
Most modern browsers come equipped with robust developer tools that can help you step through your code, inspect variables, and catch errors in real time. For instance, in Google Chrome, you can:
- Open Developer Tools by right-clicking on the page and selecting Inspect.
- Navigate to the Console tab to view JavaScript errors or logs.
- Use the Sources tab to set breakpoints and step through your code, examining variables as the script executes.
These tools allow you to identify issues more quickly and efficiently than by using console.log()
alone.
4. Error Handling with try...catch
To handle runtime errors gracefully, JavaScript provides the try...catch
statement. This allows you to "try" a block of code and "catch" any errors that occur, preventing them from crashing your entire program.
Example:
try {
let data = JSON.parse(jsonString);
} catch (error) {
console.log('Error parsing JSON:', error.message);
}
In this case, if the JSON.parse()
method fails, the catch
block will handle the error without stopping the execution of the rest of the script.
5. Debugging with Breakpoints
Breakpoints are a powerful feature available in browser developer tools. They allow you to pause the execution of your script at a specific line, inspect variable values, and control the flow of your code step by step.
To set a breakpoint in Google Chrome:
- Go to the Sources tab.
- Find the script you want to debug.
- Click on the line number where you want to set the breakpoint.
When the code execution reaches that line, it will pause, allowing you to inspect the state of the program.
6. Using the debugger
Keyword
For a more direct way to pause your code at a specific point, you can use the debugger
keyword in your JavaScript code. When the browser encounters this keyword, it will pause execution and allow you to inspect variables, just like with breakpoints.
Example:
let result = calculateSum(10, 20);
debugger; // Execution will pause here
This is particularly useful when you want to test a specific part of your code without manually setting breakpoints in the browser.
7. Third-Party Debugging Tools
In addition to browser tools, several third-party debugging tools can help you catch and fix errors in JavaScript. Popular options include:
- Sentry: A service that tracks errors in real-time and provides detailed reports on where and why they occur.
- LogRocket: A tool that records user sessions, making it easier to replicate and debug issues that occur in production.
These tools are particularly useful for larger applications or when working in teams, as they help you keep track of all errors and bugs in a central place.
8. Avoiding Common Mistakes
When debugging, it's essential to keep an eye out for common mistakes such as:
- Off-by-one errors: These occur frequently in loops and array indexing.
- Undefined or null values: Always check whether variables contain the expected values before trying to manipulate them.
- Incorrect variable scope: Be mindful of whether your variables are scoped locally or globally, as this can lead to unexpected behavior.
Conclusion
Debugging and error handling are integral parts of JavaScript development. By using techniques such as console.log()
, browser developer tools, breakpoints, and try...catch
, you can quickly identify and fix issues in your code. Additionally, leveraging third-party tools can help streamline the debugging process for more complex applications. The more proficient you become at debugging, the faster and more efficiently you can develop robust, error-free applications.
Next in the series: JavaScript Libraries: Simplifying Development with jQuery
This concludes the discussion on debugging and error handling. In the next blog, we'll dive into using jQuery, one of the most popular JavaScript libraries, to simplify DOM manipulation and streamline your web development process. Stay tuned!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript