DOM Manipulation - Interacting with HTML and CSS Using JavaScript
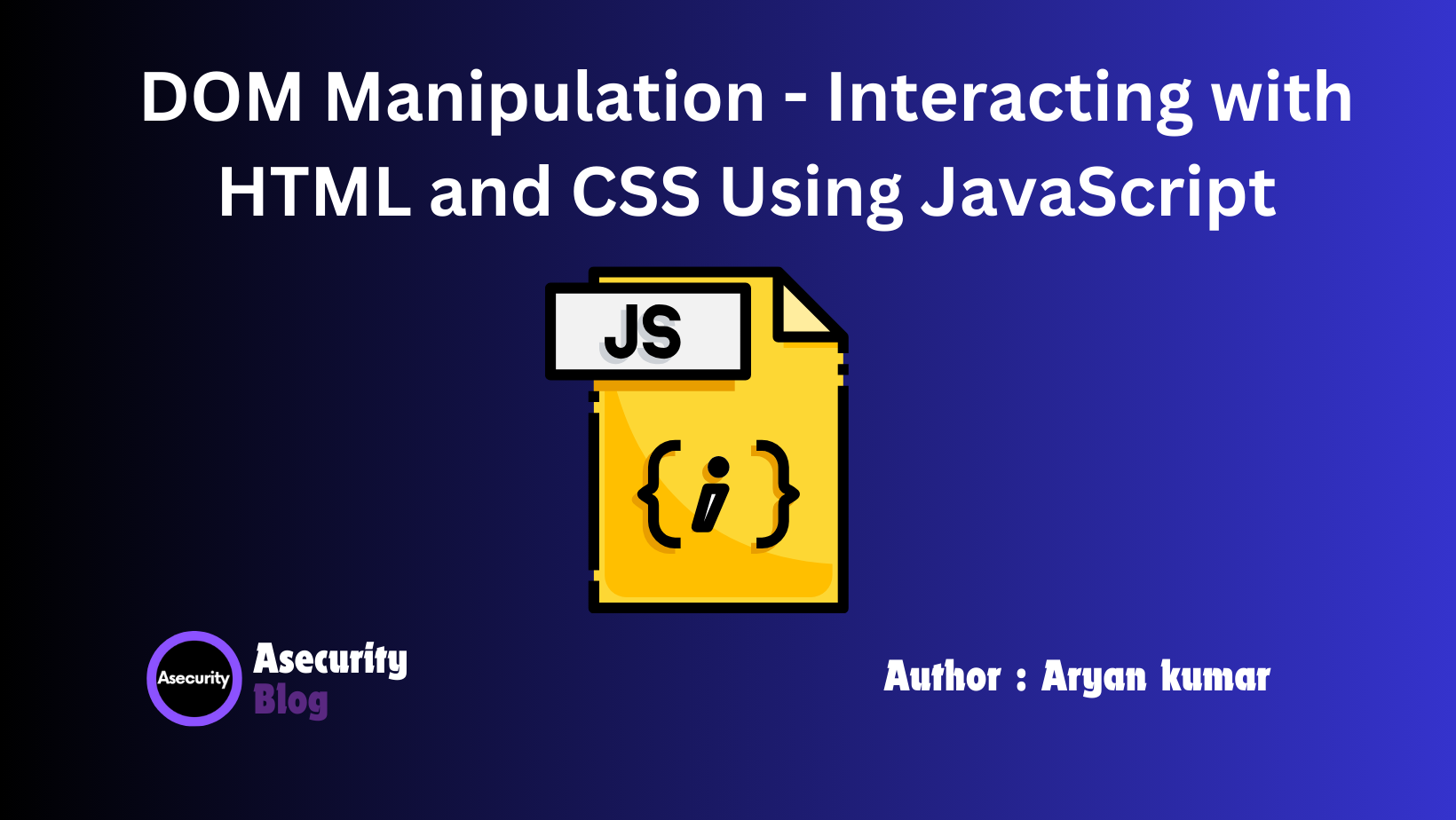
In this blog, we'll explore DOM (Document Object Model) Manipulation, a crucial skill in JavaScript that allows you to interact with HTML and CSS to create dynamic, responsive web pages. This blog will cover everything from understanding the basics of the DOM to implementing practical examples that you can try right away. Let's dive in!
What is the DOM?
The Document Object Model (DOM) is a programming interface for web documents. It represents the structure of a web page in a tree-like format, where each node is an object representing part of the document (like an element, attribute, or text). This model allows JavaScript to access, modify, and interact with the content and structure of the HTML document.
Imagine the DOM as a bridge connecting HTML and JavaScript, enabling JavaScript to dynamically alter the content, structure, and style of web pages.
How the DOM Works
When a browser loads a web page, it reads the HTML document and constructs the DOM tree. Each element in the HTML becomes a node in this tree, allowing JavaScript to target, manipulate, or even create elements dynamically.
Here's a simple HTML snippet and how the DOM represents it:
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<h1>Welcome to My Web Page</h1>
<p id="intro">This is a simple introduction to DOM manipulation.</p>
<button onclick="changeText()">Click Me</button>
</body>
</html>
The DOM tree for this HTML structure would have nodes for the <html>
, <head>
, <body>
, and other elements, each interconnected in a hierarchy that mirrors the document's structure.
Accessing the DOM with JavaScript
To interact with the DOM, JavaScript provides several methods. The most commonly used are:
document.getElementById()
: This method retrieves an element by its ID.document.querySelector()
: Selects the first element that matches a specified CSS selector.document.querySelectorAll()
: Selects all elements that match a specified CSS selector.document.createElement()
: Creates a new element in the DOM.element.appendChild()
: Adds a new child element to a parent node.
Example: Changing Text Content
Here's an example of how you can change the text content of a paragraph when a button is clicked:
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation Example</title>
</head>
<body>
<h1>DOM Manipulation with JavaScript</h1>
<p id="demo">This text will change when you click the button.</p>
<button onclick="changeText()">Change Text</button>
<script>
function changeText() {
// Accessing the element by its ID and changing its text content
document.getElementById("demo").textContent = "The text has been changed!";
}
</script>
</body>
</html>
In this example, the changeText()
function uses document.getElementById("demo")
to access the paragraph element and modify its textContent
. This is a basic yet powerful way to interact with HTML using JavaScript.
Modifying CSS with JavaScript
You can also use JavaScript to change CSS properties directly through the DOM. For instance, you can change the style of an element when an event occurs:
<!DOCTYPE html>
<html>
<head>
<title>Change Style with JavaScript</title>
</head>
<body>
<p id="styleDemo" style="color: blue;">This text color will change on click.</p>
<button onclick="changeColor()">Change Color</button>
<script>
function changeColor() {
// Accessing the element and changing its CSS style
document.getElementById("styleDemo").style.color = "red";
}
</script>
</body>
</html>
In this example, the changeColor()
function alters the CSS color
property of the paragraph with the ID styleDemo
.
Adding and Removing Elements
JavaScript allows you to add new elements to the DOM or remove existing ones, which can dynamically change the content of your web page without needing to reload.
Example: Adding a New Element
<!DOCTYPE html>
<html>
<head>
<title>DOM Add Element</title>
</head>
<body>
<ul id="list">
<li>Item 1</li>
<li>Item 2</li>
</ul>
<button onclick="addItem()">Add Item</button>
<script>
function addItem() {
// Create a new list item element
let newItem = document.createElement("li");
// Set the text content of the new element
newItem.textContent = "New Item";
// Append the new item to the list
document.getElementById("list").appendChild(newItem);
}
</script>
</body>
</html>
In this example, the addItem()
function creates a new <li>
element and adds it to the existing list.
Example: Removing an Element
<!DOCTYPE html>
<html>
<head>
<title>DOM Remove Element</title>
</head>
<body>
<div id="container">
<p>This paragraph will be removed on button click.</p>
</div>
<button onclick="removeElement()">Remove Paragraph</button>
<script>
function removeElement() {
// Access the parent element
let container = document.getElementById("container");
// Remove the first child element (the paragraph)
container.removeChild(container.firstChild);
}
</script>
</body>
</html>
This script removes a paragraph from a div
when the button is clicked, demonstrating how JavaScript can dynamically alter the document structure.
Conclusion
Mastering DOM manipulation allows you to create highly interactive and responsive web applications. By learning to access, modify, and manipulate HTML and CSS through JavaScript, you can elevate your web development skills to the next level. Practice these examples and experiment with the DOM to gain a deeper understanding of how JavaScript can enhance your web pages.
In the next blog, we will dive into handling user actions with JavaScript by responding to various events, making your web applications even more interactive!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript