Handling User Actions: Responding to Events with JavaScript
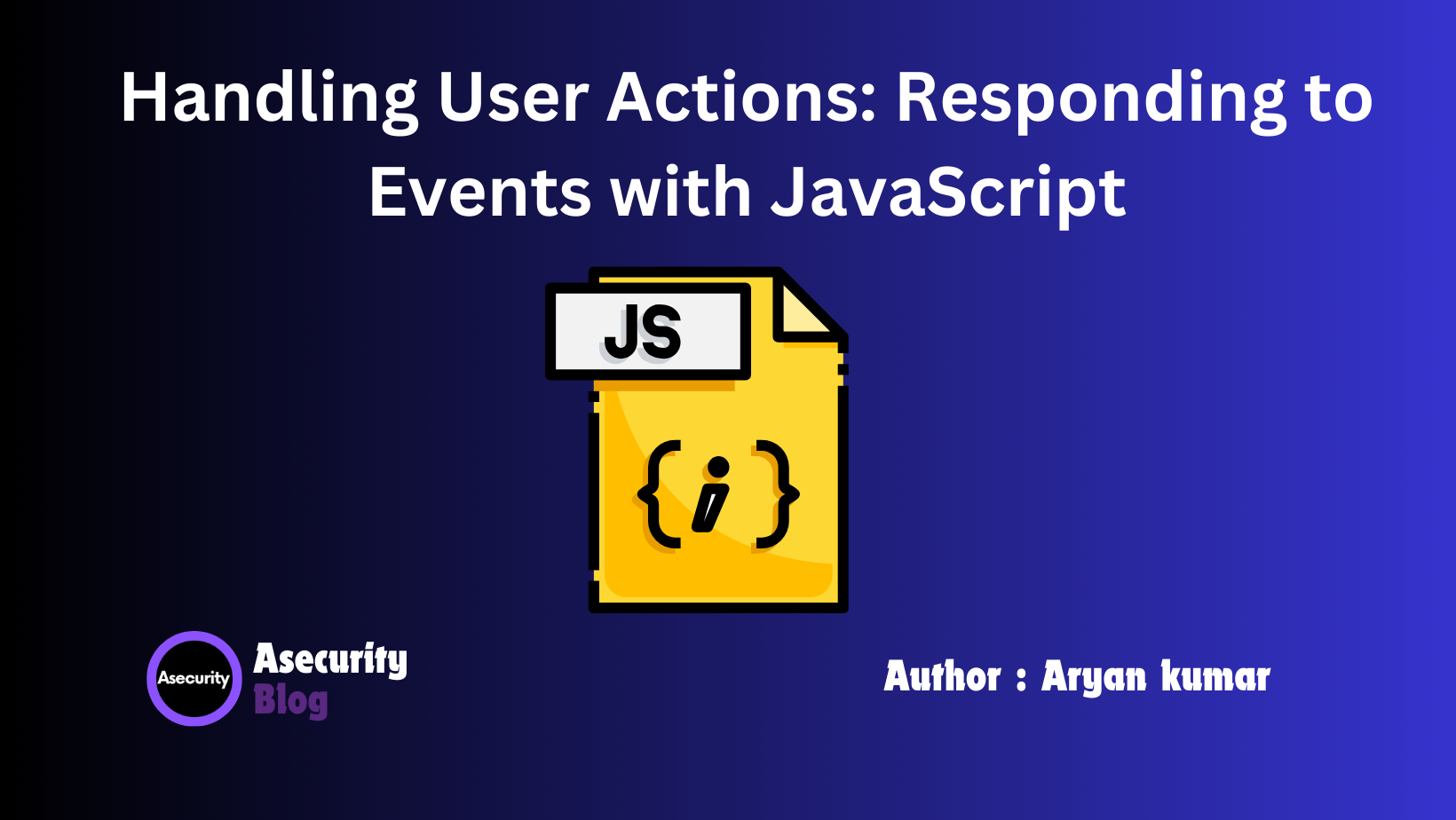
In this blog, we will explore how to handle user actions using JavaScript, a crucial skill for making web pages interactive. If you're new to JavaScript, don't worry! We'll break down the concepts step-by-step so you can understand how to respond to events like clicks, key presses, and more.
What Are Events in JavaScript?
Events are actions or occurrences that happen in the browser, like clicking a button, submitting a form, or pressing a key. JavaScript allows us to detect these events and respond to them, making our web pages dynamic and interactive.
Common Types of Events
- Mouse Events: Actions triggered by the mouse, such as:
click
: When the user clicks on an element.dblclick
: When the user double-clicks on an element.mouseover
: When the mouse pointer moves over an element.mouseout
: When the mouse pointer moves out of an element.
- Keyboard Events: Actions triggered by keyboard inputs, such as:
keydown
: When a key is pressed down.keyup
: When a key is released.keypress
: When a key is pressed and released.
- Form Events: Actions that occur in forms, such as:
submit
: When a form is submitted.change
: When the value of a form element changes.focus
: When an element gains focus.
- Window Events: Actions triggered in the browser window, such as:
load
: When the page finishes loading.resize
: When the browser window is resized.scroll
: When the user scrolls the page.
Adding Event Listeners
An event listener is a function that waits for a specific event to occur and then executes a block of code. You can add an event listener to any HTML element using the addEventListener()
method. Here's a basic syntax:
element.addEventListener('event', function);
element
: The HTML element to which the listener is added (e.g., a button).event
: The type of event to listen for (e.g.,'click'
).function
: The code to run when the event happens.
Example: Handling Click Events
Let’s create a simple button that changes color when clicked.
HTML:
<button id="colorButton">Click Me!</button>
JavaScript:
// Select the button element
const button = document.getElementById('colorButton');
// Add a click event listener
button.addEventListener('click', function() {
// Change the button's background color
button.style.backgroundColor = 'blue';
});
In this example:
- We first select the button using
document.getElementById()
. - We then add an event listener that listens for a
'click'
event. - When the button is clicked, the background color changes to blue.
Handling Form Submission Events
Forms are crucial for collecting user input, and handling form submission is a common task. Let’s see how to validate a form before submission.
HTML:
<form id="myForm">
<input type="text" id="username" placeholder="Enter your username">
<button type="submit">Submit</button>
</form>
JavaScript:
// Select the form element
const form = document.getElementById('myForm');
// Add a submit event listener
form.addEventListener('submit', function(event) {
// Prevent the form from submitting
event.preventDefault();
// Get the input value
const username = document.getElementById('username').value;
// Check if the username is empty
if (username === '') {
alert('Username cannot be empty!');
} else {
alert('Form submitted successfully!');
}
});
In this example:
- We prevent the form from submitting using
event.preventDefault()
to validate the input first. - We check if the username field is empty. If it is, we alert the user; otherwise, we allow the submission.
Best Practices for Handling Events
- Use
addEventListener()
: It’s more flexible and allows multiple event listeners on the same element compared to inline event handlers likeonclick
. - Prevent Default Behavior When Necessary: Use
event.preventDefault()
to stop the default action of events, like preventing form submission for validation purposes. - Clean Up Event Listeners: Remove event listeners when they are no longer needed using
removeEventListener()
to improve performance.
Conclusion
Handling user actions is key to creating interactive web experiences. By learning how to respond to events with JavaScript, you can build responsive and dynamic web applications that respond to user input effortlessly. Experiment with different event types and handlers to enhance your skills further. Keep practicing, and you'll soon master the art of event-driven programming!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript