HTML Best Practices: Writing Clean, Maintainable, and Accessible Code
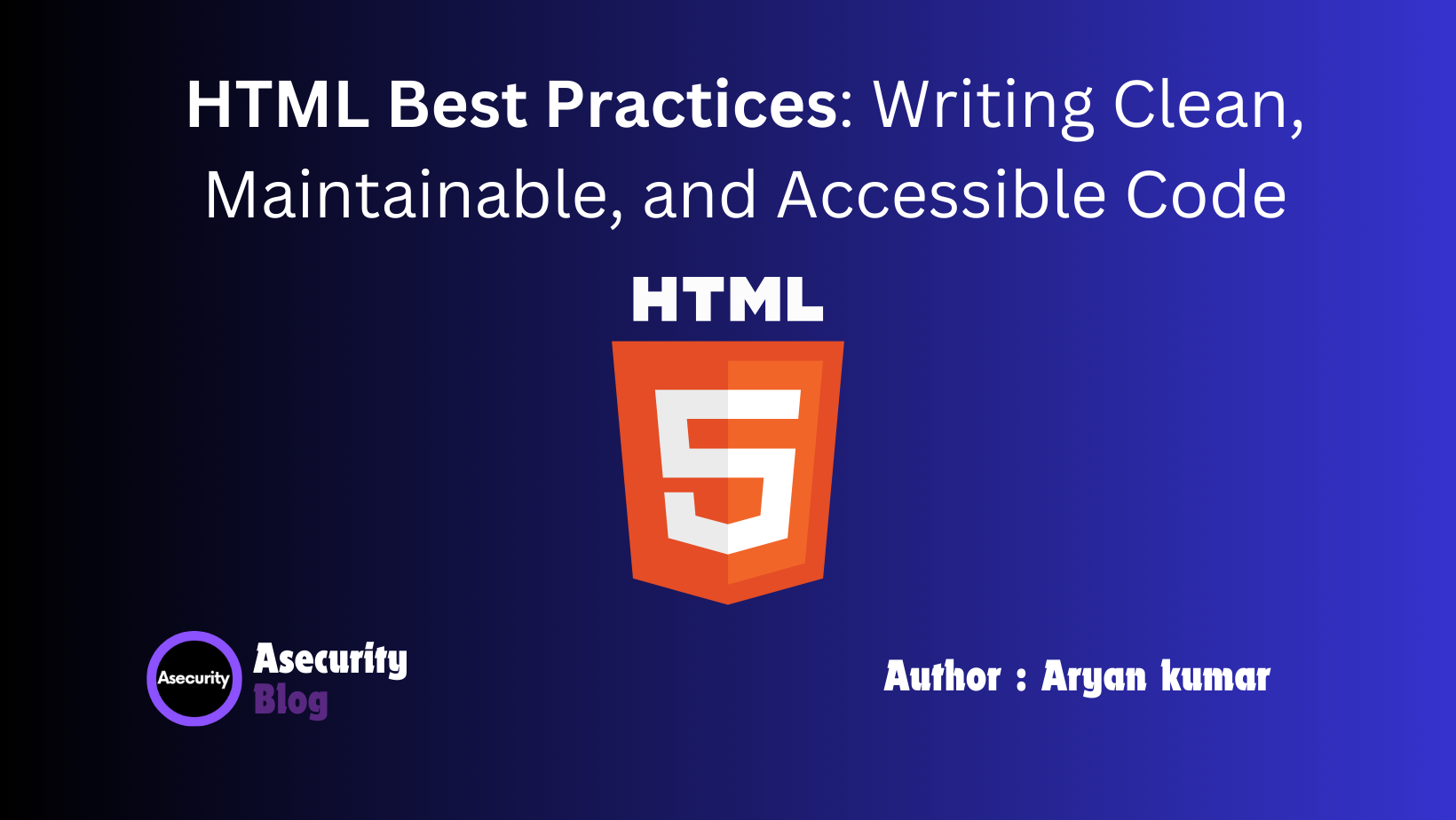
As you continue to develop your skills in HTML, it’s essential to not only focus on functionality but also on writing code that is clean, maintainable, and accessible. Good coding practices ensure that your web pages are easy to read, debug, and enhance over time, and that they are accessible to all users, including those with disabilities. In this blog, we'll explore some of the best practices in HTML that will help you create professional-quality websites.
1. Writing Clean and Readable Code
Clean code is the foundation of any good website. It makes your HTML documents easier to understand, both for you and others who may work on the project in the future.
Use Proper Indentation: Indentation helps in structuring your HTML code, making it easier to read. For example:
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a paragraph of text.</p>
</body>
</html>
Keep Code DRY (Don’t Repeat Yourself): Avoid repeating code unnecessarily. Reusing code not only saves time but also makes your HTML more efficient and easier to update.
Comment Your Code: Use comments to explain sections of your code. This is particularly helpful when you or others revisit the code later. Comments are added using <!-- -->
:
<!-- This is a comment -->
<p>This is a paragraph.</p>
2. Ensuring Maintainability
As your projects grow in complexity, maintainability becomes crucial. This means writing HTML that can be easily updated and modified.
Use Consistent Naming Conventions: When naming classes, IDs, and other elements, consistency is key. Use descriptive names that make it clear what each element is for, and stick to a consistent pattern throughout your project.
For example, if you're naming classes for a navigation menu:
<nav class="main-nav">
<ul class="nav-list">
<li class="nav-item"><a href="#">Home</a></li>
<li class="nav-item"><a href="#">About</a></li>
</ul>
</nav>
Separate Structure and Presentation: Keep your HTML for structure and CSS for presentation. Avoid using inline styles or JavaScript within your HTML files. This separation makes it easier to maintain and update your code.
3. Writing Accessible HTML
Accessibility ensures that all users, including those with disabilities, can interact with your web content effectively. HTML offers various elements and attributes that enhance accessibility.
Use Semantic HTML: Semantic elements like <header>
, <nav>
, <article>
, and <footer>
provide meaning to the content structure and help screen readers navigate your page more effectively.
<header>
<h1>Website Title</h1>
</header>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
</ul>
</nav>
<article>
<h2>Article Title</h2>
<p>This is an article.</p>
</article>
<footer>
<p>Footer information</p>
</footer>
Use alt
Attributes for Images: Always include alt
attributes for images to describe their content. This is crucial for users who rely on screen readers.
<img src="image.jpg" alt="Description of the image">
Ensure Keyboard Accessibility: Ensure that all interactive elements (like links and buttons) are accessible via the keyboard. This involves using HTML elements that are naturally focusable or can be made focusable using the tabindex
attribute.
Label Your Forms: When creating forms, use <label>
elements to ensure that each input field is properly associated with its label, enhancing usability for screen readers.
<label for="email">Email:</label>
<input type="email" id="email" name="email">
4. Testing and Validation
After writing your HTML, it's essential to test and validate it to ensure there are no errors and that it meets web standards.
Use HTML Validators: Validators like the W3C Markup Validation Service can help identify errors and enforce best practices.
Cross-Browser Testing: Test your HTML in different browsers (e.g., Chrome, Firefox, Safari, Edge) to ensure it displays consistently.
Accessibility Testing: Use tools like WAVE or Lighthouse to check the accessibility of your web pages and make necessary adjustments.
Conclusion
By following these best practices, you'll not only create web pages that work well today but also ensure they are easy to maintain and update in the future. Writing clean, maintainable, and accessible HTML is a key step towards becoming a proficient web developer.
In the next part of our journey, we will dive into the world of CSS, where you'll learn how to style your HTML documents, bringing your web pages to life with color, fonts, and layout techniques. Stay tuned as we take the next step in mastering web development
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript