Interactive Forms: Collecting and Managing User Input with HTML
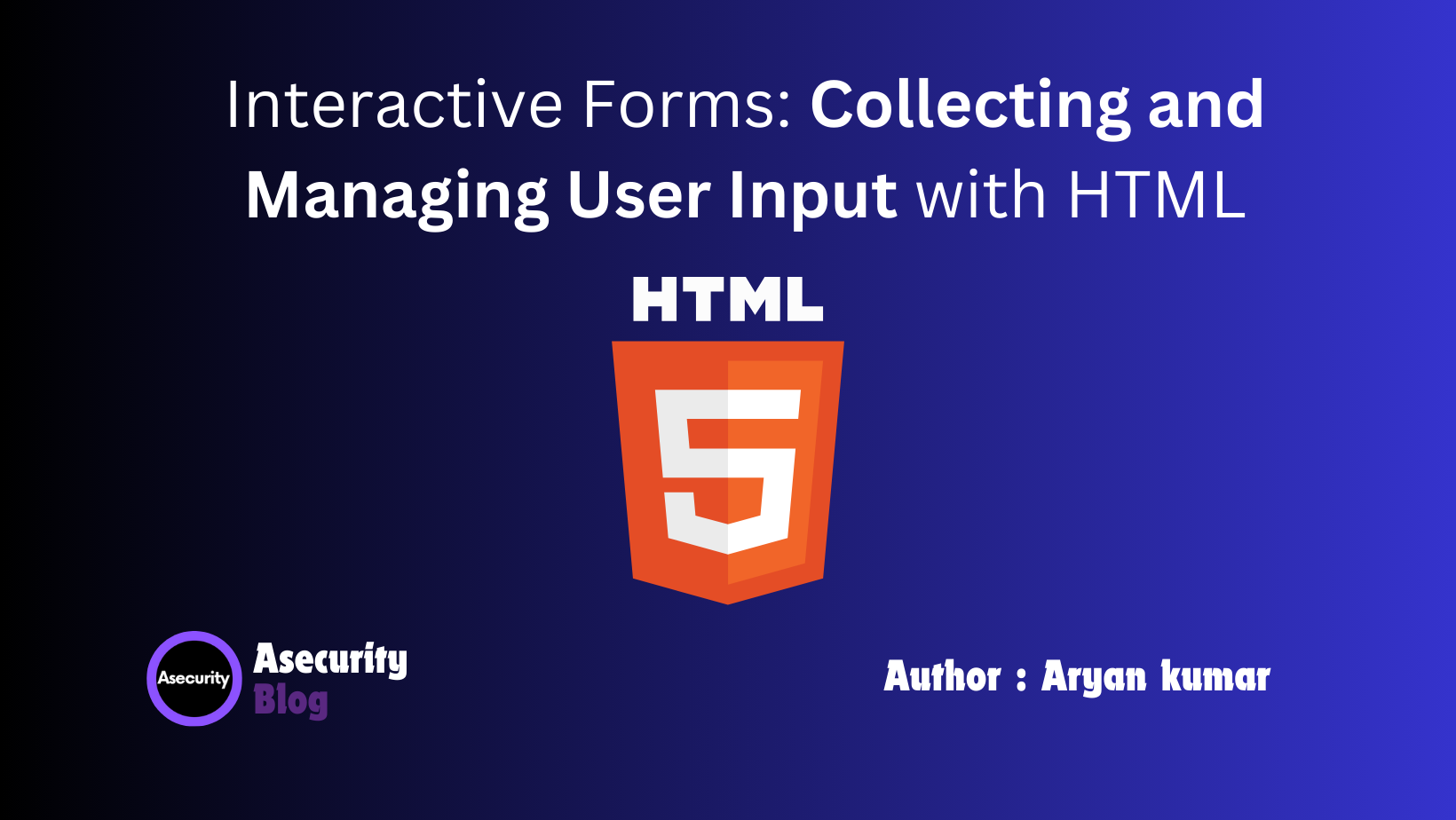
Welcome back to our ongoing series, "Mastering Web Development with HTML, CSS, and JavaScript." In our previous discussions, we laid the foundation by exploring the basics of HTML, guiding you through creating your first HTML document, and diving into essential HTML tags and elements. Today, we continue our journey by delving into a crucial aspect of web development: building interactive forms with HTML.
The Power of Interactive Forms
Forms are the backbone of user interaction on the web. They allow users to input data, submit queries, and engage with your website in a meaningful way. Whether you're creating a simple contact form, a survey, or a complex registration system, understanding how to construct and manage forms is essential for any web developer.
Key Components of HTML Forms
Before we start building forms, let's review the core components that make up an HTML form:
- Form Element: This is the container for all form elements. It defines how data is sent and processed.
<form action="/submit-form" method="post">
</form>
- Input Elements: These are used to collect data from users. Common types include text, email, password, and checkbox.
<input type="text" name="username" placeholder="Enter your username">
<input type="email" name="email" placeholder="Enter your email">
<input type="password" name="password" placeholder="Enter your password">
- Labels: Labels improve accessibility by describing each form element, making it easier for screen readers to interpret.
<label for="username">Username:</label>
<input type="text" id="username" name="username">
- Buttons: These enable users to submit the form or reset their input.
<button type="submit">Submit</button>
<button type="reset">Reset</button>
Building Your First Interactive Form
Let's create a simple contact form that includes fields for the user's name, email, message, and a submit button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Us</title>
</head>
<body>
<h2>Contact Us</h2>
<form action="/submit-form" method="post">
<div>
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
</div>
<div>
<label for="message">Message:</label>
<textarea id="message" name="message" rows="4" required></textarea>
</div>
<button type="submit">Send Message</button>
</form>
</body>
</html>
Enhancing Form Accessibility and Usability
- Accessibility: Ensure every input has a corresponding
<label>
. This not only aids visually impaired users but also enhances the overall user experience. - Validation: Use HTML5 validation attributes like
required
,minlength
, andmaxlength
to guide users and ensure data integrity. - Styling: Use CSS to make your form visually appealing and consistent with your website's design. Ensure buttons are easily clickable, and input fields are clearly distinguishable.
Managing Form Data
Once a form is submitted, the data needs to be handled effectively. Typically, form data is sent to a server for processing. This can be done using the POST
method, which securely sends data to the server.
Server-Side Processing
In a server-side language like PHP, you can capture the submitted form data as follows:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = htmlspecialchars($_POST['name']);
$email = htmlspecialchars($_POST['email']);
$message = htmlspecialchars($_POST['message']);
// Process the data (e.g., save to a database, send an email)
}
?>
Conclusion
Building interactive forms is a vital skill in web development that enhances user interaction and data collection. By understanding the components of HTML forms and how to manage form data, you can create engaging and functional user experiences.
In our next blog post, we'll explore how to enhance the structure and accessibility of your web pages using HTML5 semantic elements. Stay tuned as we continue to unlock the potential of web development with HTML, CSS, and JavaScript.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript