JavaScript 101: Introduction to Adding Interactivity to Your Web Pages
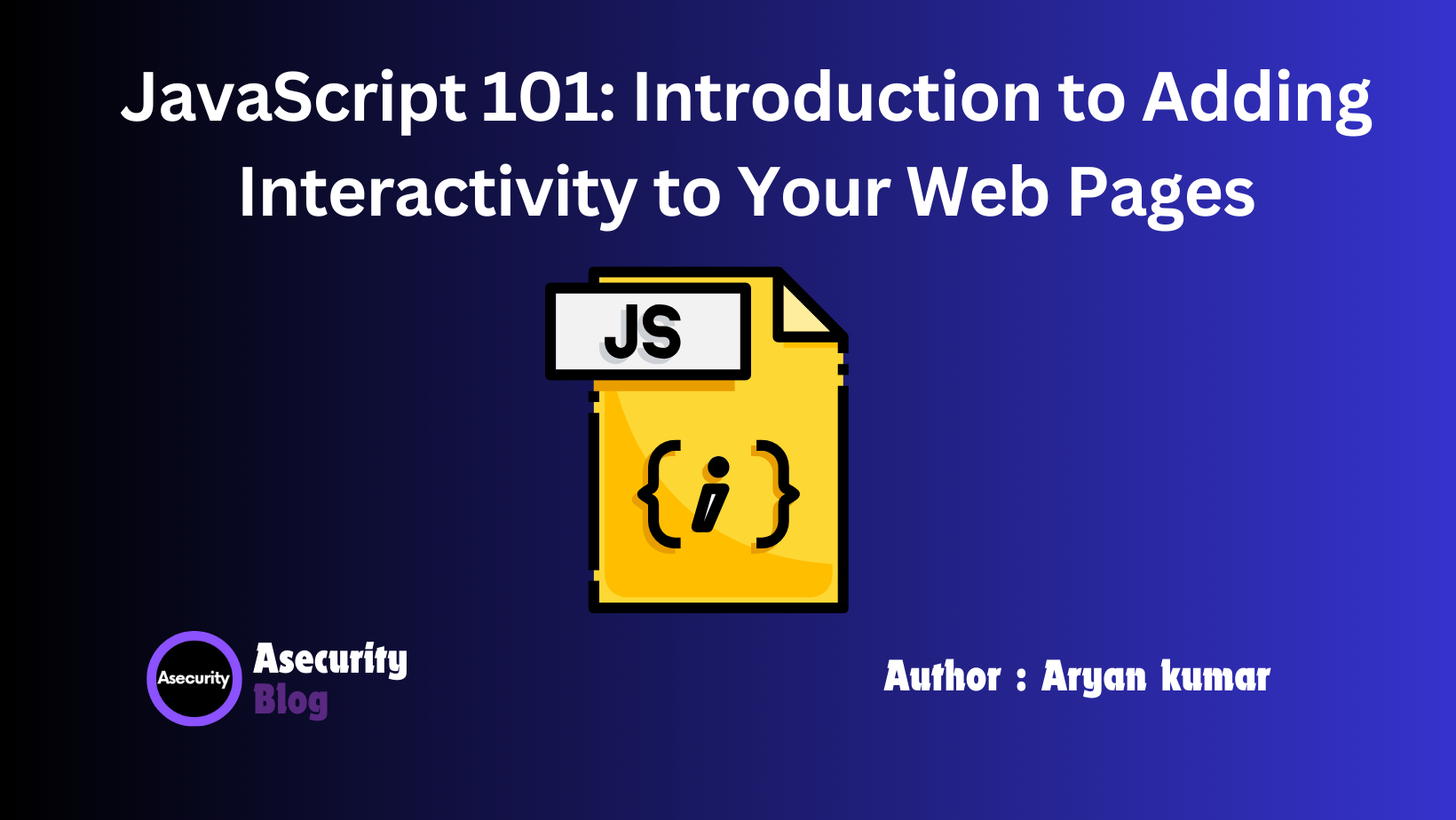
JavaScript is the magic behind interactive web pages. While HTML structures your content and CSS styles it, JavaScript brings everything to life. In this beginner’s guide, we’ll introduce you to the basics of JavaScript, giving you a taste of how it can elevate your web development skills. By the end, you'll realize how our course provides a solid foundation for mastering this powerful language.
Why JavaScript?
Ever wondered how websites respond to your actions without needing to reload the page? That’s JavaScript at work! Whether you're clicking a button, filling out a form, or seeing live data updates, JavaScript enables websites to react in real-time. It's what makes the web feel dynamic and engaging.
JavaScript’s versatility is what makes it one of the most popular programming languages. From controlling multimedia to building complex web applications, learning JavaScript opens up endless possibilities for your web projects.
Embedding JavaScript into Your Web Pages
To get started, the first step is to embed JavaScript into your HTML file. It’s simpler than you think! You can either add JavaScript directly in your HTML document using the <script>
tag or link to an external JavaScript file for better organization. Here’s how:
Inline JavaScript (Directly in HTML):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript 101</title>
</head>
<body>
<h1>Welcome to JavaScript!</h1>
<button onclick="greet()">Click Me</button>
<script>
function greet() {
alert('Hello, JavaScript is working!');
}
</script>
</body>
</html>
In this example, clicking the button will trigger a simple JavaScript function that displays an alert message. This is just the beginning of what you can do!
External JavaScript (Best Practice for Larger Projects)
As your projects grow, it’s a good idea to keep your JavaScript in separate files for better organization and readability.
Here’s how you would link to an external JavaScript file:
HTML File:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript 101</title>
</head>
<body>
<h1>Welcome to JavaScript!</h1>
<button onclick="greet()">Click Me</button>
<script src="script.js"></script>
</body>
</html>
External JavaScript File (script.js
):
function greet() {
alert('Hello, from external JavaScript!');
}
With this structure, you’ll have a cleaner codebase, making it easier to maintain and scale as you add more features.
How JavaScript Enhances User Interaction
Now that you know how to include JavaScript in your pages, let’s explore why it’s so important. JavaScript is the backbone of user interaction on the web. For example:
- Form Validation: You can use JavaScript to ensure that users fill out forms correctly before submitting.
- Dynamic Content: With JavaScript, you can change or update content without reloading the page, making your site feel more responsive.
- Animations: Want to add subtle animations to buttons, menus, or entire pages? JavaScript allows you to do that effortlessly.
Your First Steps Towards JavaScript Mastery
Understanding the basics of JavaScript is the first step to unlocking its full potential. As you dive deeper, you’ll discover how JavaScript can control everything from interactive forms to complex applications.
In our full course, we’ll guide you through the journey, ensuring you gain not just theoretical knowledge, but also hands-on experience. You’ll learn how to:
- Work with variables, data types, and operators
- Write reusable functions to streamline your code
- Use conditionals and loops to control logic flow
- Manipulate the DOM to interact with HTML and CSS in real-time
Each concept is broken down with easy-to-understand examples, ensuring you don’t just learn JavaScript—you master it.
Ready to Get Started?
This is just the beginning of your JavaScript journey. Stay tuned as we dive into more topics like handling user events, DOM manipulation, and making your web pages smarter and more responsive.
With our course, you won’t just follow along—you’ll build interactive, real-world web applications that showcase your skills. This is your chance to learn JavaScript in a way that’s practical, hands-on, and exciting.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript