JavaScript Libraries: Simplifying Development with jQuery
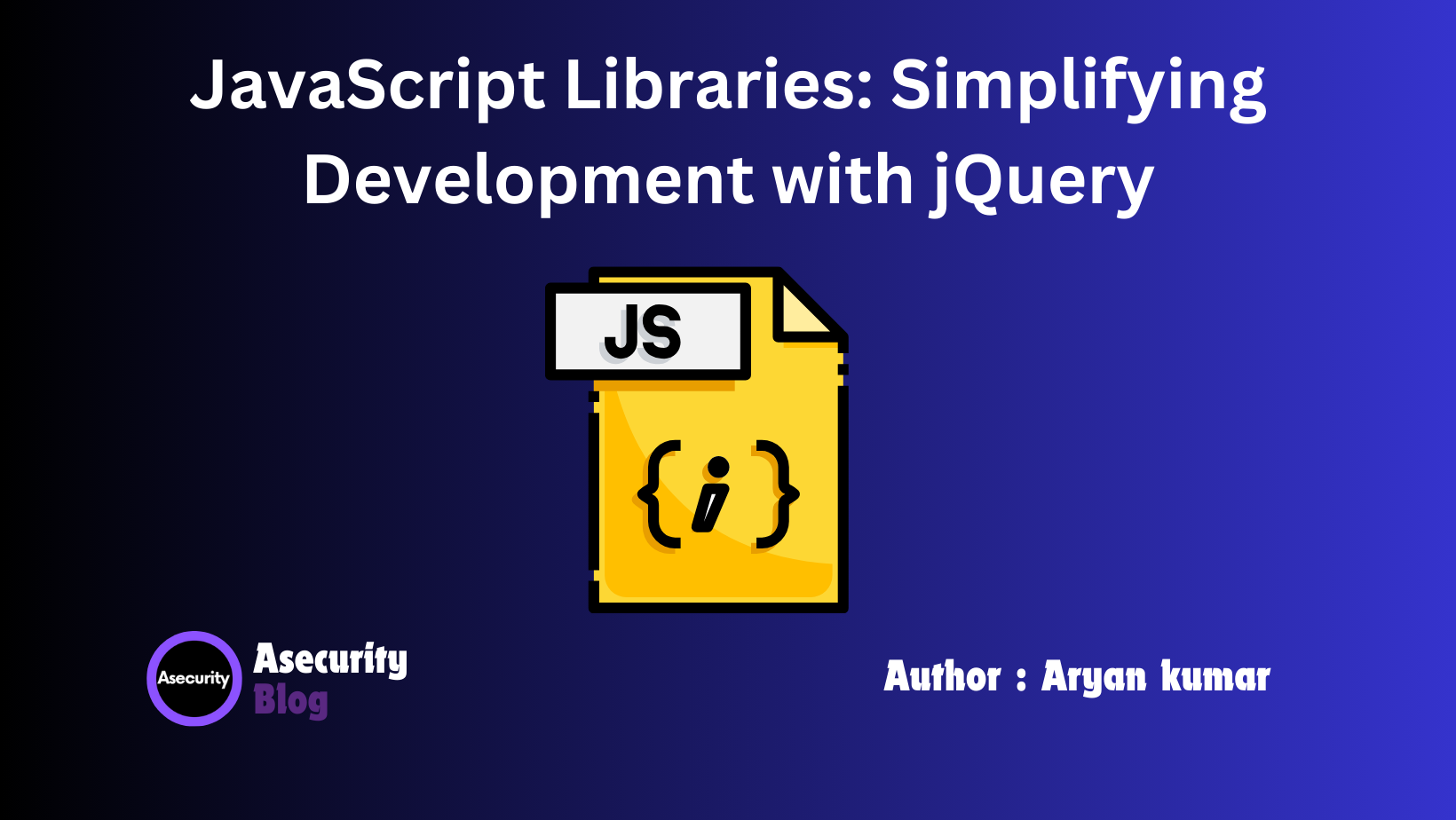
JavaScript has evolved significantly over the years, and while modern JavaScript (ES6 and beyond) provides powerful capabilities, libraries like jQuery still play a significant role in simplifying web development. jQuery, once the go-to library for making JavaScript easier to use, offers a streamlined API that makes DOM manipulation, event handling, and AJAX calls much simpler.
In this blog, we’ll explore how jQuery can help you simplify development and enhance your productivity. Even though many modern JavaScript features have reduced the dependency on jQuery, it remains a valuable tool for quickly building dynamic web pages with minimal code.
1. What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies tasks such as DOM manipulation, event handling, animation, and AJAX calls. The primary goal of jQuery is to make it easier to write cross-browser compatible JavaScript code.
Here’s an example of how jQuery simplifies common tasks:
<!-- Without jQuery -->
document.getElementById('myElement').style.color = 'red';
<!-- With jQuery -->
$('#myElement').css('color', 'red');
As you can see, jQuery allows you to write less code to achieve the same result, making your development process faster and more efficient.
2. Getting Started with jQuery
Before you can start using jQuery, you need to include the library in your HTML file. You can either download jQuery or link it via a CDN (Content Delivery Network). Here’s how to include it using a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once included, you can start using jQuery by wrapping your code in a $(document).ready()
function to ensure your code runs after the DOM has fully loaded:
$(document).ready(function() {
// Your jQuery code goes here
});
3. Simplifying DOM Manipulation
One of the most common tasks in JavaScript is manipulating the DOM (Document Object Model). With jQuery, DOM manipulation becomes more concise and readable. Some common tasks include selecting elements, changing content, and modifying CSS properties.
- Selecting Elements: jQuery uses CSS-style selectors, making it easier to target elements.
// Select elements with a class of 'btn'
$('.btn').hide(); // Hides all elements with the class 'btn'
- Modifying Content: jQuery allows you to change the text, HTML, or attributes of an element with simple methods.
$('#title').text('New Title'); // Changes the text of the element with ID 'title'
- Changing CSS Styles: With the
.css()
method, you can apply multiple CSS properties to elements in one go.
$('#box').css({
'background-color': 'blue',
'width': '200px',
'height': '100px'
});
4. Handling Events with Ease
Handling user events like clicks, mouse movements, or key presses is a breeze with jQuery. The .on()
method attaches event handlers to elements, allowing you to respond to user interactions in an elegant way.
For example, here’s how you can handle a button click event:
$('#submitBtn').on('click', function() {
alert('Button clicked!');
});
This simplifies event handling compared to native JavaScript, which would require more code for cross-browser compatibility.
5. Animations in jQuery
jQuery makes adding animations to your web page simple. With built-in methods like .fadeIn()
, .fadeOut()
, .slideUp()
, and .slideDown()
, you can create smooth animations without needing external libraries.
For example, to fade in an element when a button is clicked:
$('#fadeInBtn').on('click', function() {
$('#box').fadeIn('slow');
});
This feature is especially useful for creating dynamic and interactive user interfaces.
6. AJAX Made Simple
One of jQuery’s most powerful features is its ability to handle AJAX (Asynchronous JavaScript and XML) requests. AJAX allows you to fetch data from a server and update your web page without reloading it. jQuery’s .ajax()
method makes it simple to send asynchronous HTTP requests.
Here’s an example of a basic AJAX call:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
$('#content').html(response);
},
error: function() {
alert('An error occurred');
}
});
This reduces the complexity of making HTTP requests and processing responses, allowing you to focus more on the logic of your web app rather than the intricacies of the request.
7. Cross-Browser Compatibility
One of the major reasons for jQuery’s popularity was its ability to abstract away browser inconsistencies. Writing cross-browser compatible code was difficult before modern standards were widely adopted, but jQuery smoothed over many of the differences. Although modern JavaScript has made this less of a concern, jQuery is still useful when supporting older browsers.
8. Downsides of jQuery
While jQuery offers many advantages, it’s essential to consider some potential downsides:
- File Size: Although small by itself, adding jQuery to your project can increase the overall size of your site if you don’t need its full functionality.
- Learning Curve: For beginners unfamiliar with the basics of JavaScript, learning jQuery might be overwhelming, especially since modern JavaScript can often achieve the same results.
- Redundancy: Many tasks that once required jQuery can now be accomplished with modern JavaScript (ES6+) in fewer lines, without needing an external library.
9. When Should You Use jQuery?
Despite the rise of modern JavaScript frameworks like React and Vue, jQuery still holds value, especially in the following cases:
- Legacy Projects: If you’re working on a project that already uses jQuery, it’s more efficient to continue using it rather than rewriting everything in modern JavaScript.
- Quick Prototyping: When you need to quickly build a prototype or add dynamic behavior to a static website, jQuery can speed up the process.
- Simple Projects: If your project doesn’t require complex state management or advanced features, jQuery might be all you need.
Conclusion
jQuery continues to be a useful tool for web developers, offering simplicity and power for common JavaScript tasks. Whether you’re manipulating the DOM, handling events, or making AJAX requests, jQuery can save you time and effort with its concise and cross-browser compatible code. However, it’s essential to evaluate whether jQuery is the best choice for your project, especially with the rise of modern JavaScript alternatives.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript