Optimizing JavaScript for Performance: Best Practices and Tips
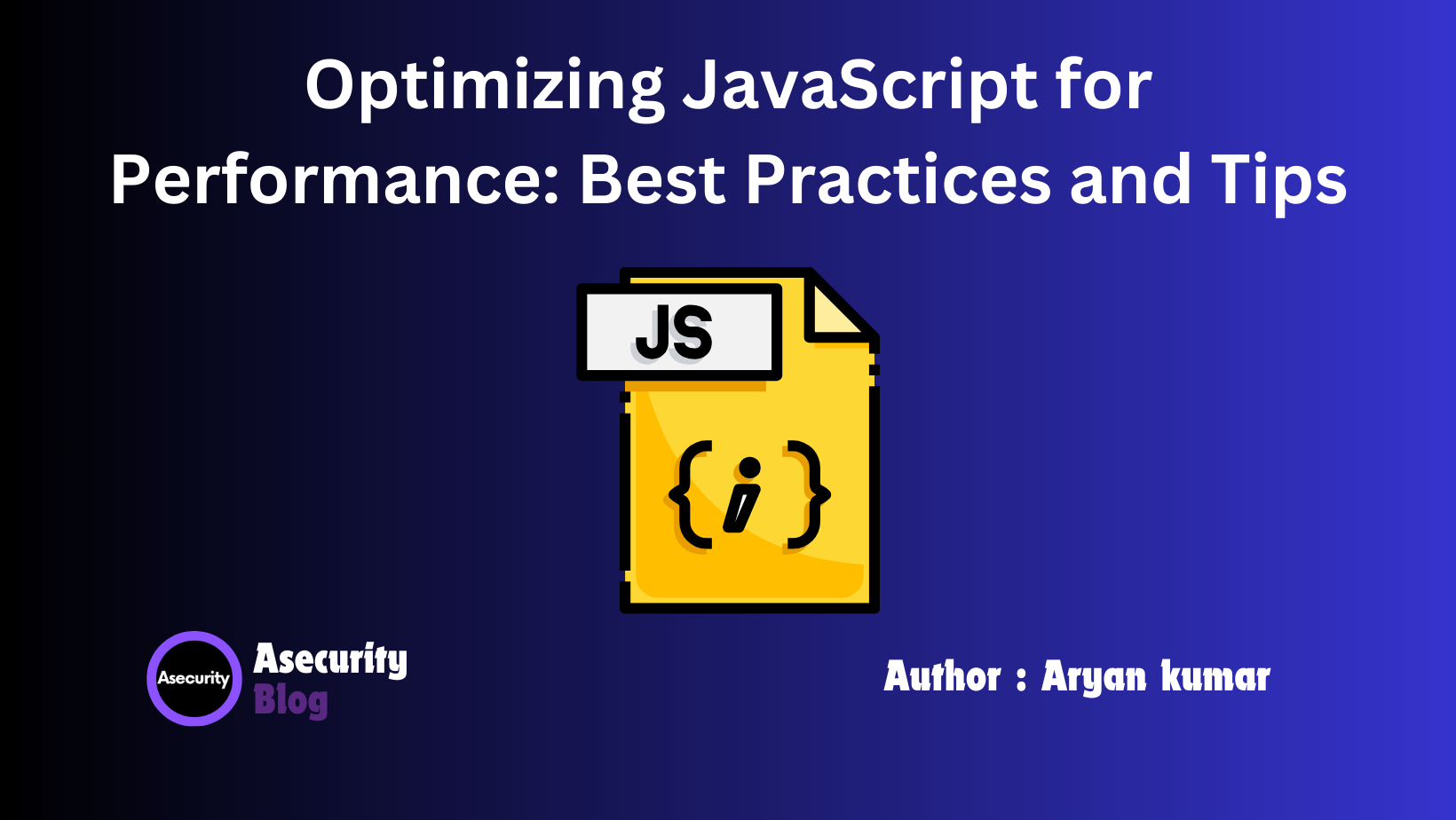
When working with JavaScript, especially in large-scale applications, performance becomes a crucial factor in maintaining smooth user experiences. As web pages grow more interactive, the demand for optimized code increases to avoid performance bottlenecks. In this blog, we’ll dive into practical tips and techniques for optimizing JavaScript, ensuring your code runs efficiently.
1. Minimize DOM Access
The DOM (Document Object Model) is the bridge between JavaScript and HTML, allowing manipulation of page content. However, excessive DOM access can slow down performance. Each time your JavaScript interacts with the DOM, the browser must recalculate styles and reflow the page, which can be costly in terms of performance.
Tip:
- Batch DOM Changes: Instead of making multiple individual changes, group them together. For example, modify multiple properties or attributes of an element at once.
- Use Document Fragments: When adding multiple elements to the DOM, consider using document fragments. It prevents multiple reflows and repaints.
let fragment = document.createDocumentFragment();
for (let i = 0; i < 100; i++) {
let newElement = document.createElement('div');
fragment.appendChild(newElement);
}
document.body.appendChild(fragment);
2. Avoid Memory Leaks
Memory leaks occur when memory that’s no longer needed is not released, leading to performance degradation. In JavaScript, memory management is handled automatically via garbage collection. However, certain coding patterns can prevent memory from being properly released.
Tip:
- Avoid Global Variables: Global variables persist throughout the lifetime of the page, increasing the chances of memory leaks. Keep variables in local scopes whenever possible.
- Clean Up Event Listeners: Event listeners attached to DOM elements should be removed when the element is no longer in use. Unremoved event listeners can cause memory leaks.
function handleClick() {
console.log('Button clicked');
}
const button = document.getElementById('myButton');
button.addEventListener('click', handleClick);
// Remove the event listener when it's no longer needed
button.removeEventListener('click', handleClick);
3. Debounce and Throttle Event Listeners
Handling events like scrolling, resizing, or keypresses can trigger numerous function calls in a short period, putting a heavy load on your application. To mitigate this, implement debounce and throttle techniques to limit how often your event handlers are executed.
Debouncing: Limits the execution of a function by waiting for a period of inactivity before running it.
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => func.apply(this, args), delay);
};
}
window.addEventListener('resize', debounce(() => {
console.log('Window resized');
}, 200));
Throttling: Ensures a function is executed at most once in a given time interval, regardless of how many events occur.
function throttle(func, limit) {
let inThrottle;
return function (...args) {
if (!inThrottle) {
func.apply(this, args);
inThrottle = true;
setTimeout(() => (inThrottle = false), limit);
}
};
}
window.addEventListener('scroll', throttle(() => {
console.log('Scrolling');
}, 100));
4. Lazy Load Resources
Not all resources need to be loaded immediately when a page loads. Lazy loading delays the loading of non-critical resources (e.g., images, scripts) until they are needed.
Tip:
- Lazy Load Images: Only load images as they come into the viewport, reducing the initial page load time.
<img src="placeholder.jpg" data-src="image.jpg" class="lazyload">
<script>
const images = document.querySelectorAll('img.lazyload');
const lazyLoad = (image) => {
const src = image.getAttribute('data-src');
image.src = src;
image.classList.remove('lazyload');
};
images.forEach((img) => {
if (img.getBoundingClientRect().top < window.innerHeight) {
lazyLoad(img);
}
});
window.addEventListener('scroll', () => {
images.forEach((img) => {
if (img.getBoundingClientRect().top < window.innerHeight) {
lazyLoad(img);
}
});
});
</script>
5. Use Asynchronous Code Wisely
Asynchronous programming allows certain tasks to run in the background without blocking the main thread. JavaScript’s async/await
and Promises make it easier to handle asynchronous operations, but it's essential to ensure that you're using them efficiently.
Tip:
- Use
async/await
for Readability: Convert nested callbacks into cleanerasync/await
code.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
Parallelize Independent Tasks: When you have multiple independent asynchronous tasks, run them in parallel to save time.
async function fetchMultiple() {
const [data1, data2] = await Promise.all([
fetch('https://api.example.com/data1'),
fetch('https://api.example.com/data2')
]);
console.log(data1, data2);
}
6. Optimize Loops
Loops are fundamental to any programming language, but poorly optimized loops can significantly slow down your code.
Tip:
- Minimize DOM Lookups in Loops: Cache references to DOM elements outside the loop.
- Use
forEach
andmap
: These array methods are more optimized and easier to read than traditionalfor
loops.
const elements = document.querySelectorAll('.item');
elements.forEach((element) => {
element.style.color = 'blue';
});
Conclusion
Optimizing JavaScript for performance is all about writing clean, efficient code and minimizing unnecessary overhead. By following these best practices, you can ensure your web applications run faster and provide a seamless user experience. Keep experimenting, profiling, and fine-tuning your code as you build more complex applications.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript