Rate Limiting In Modern APIs
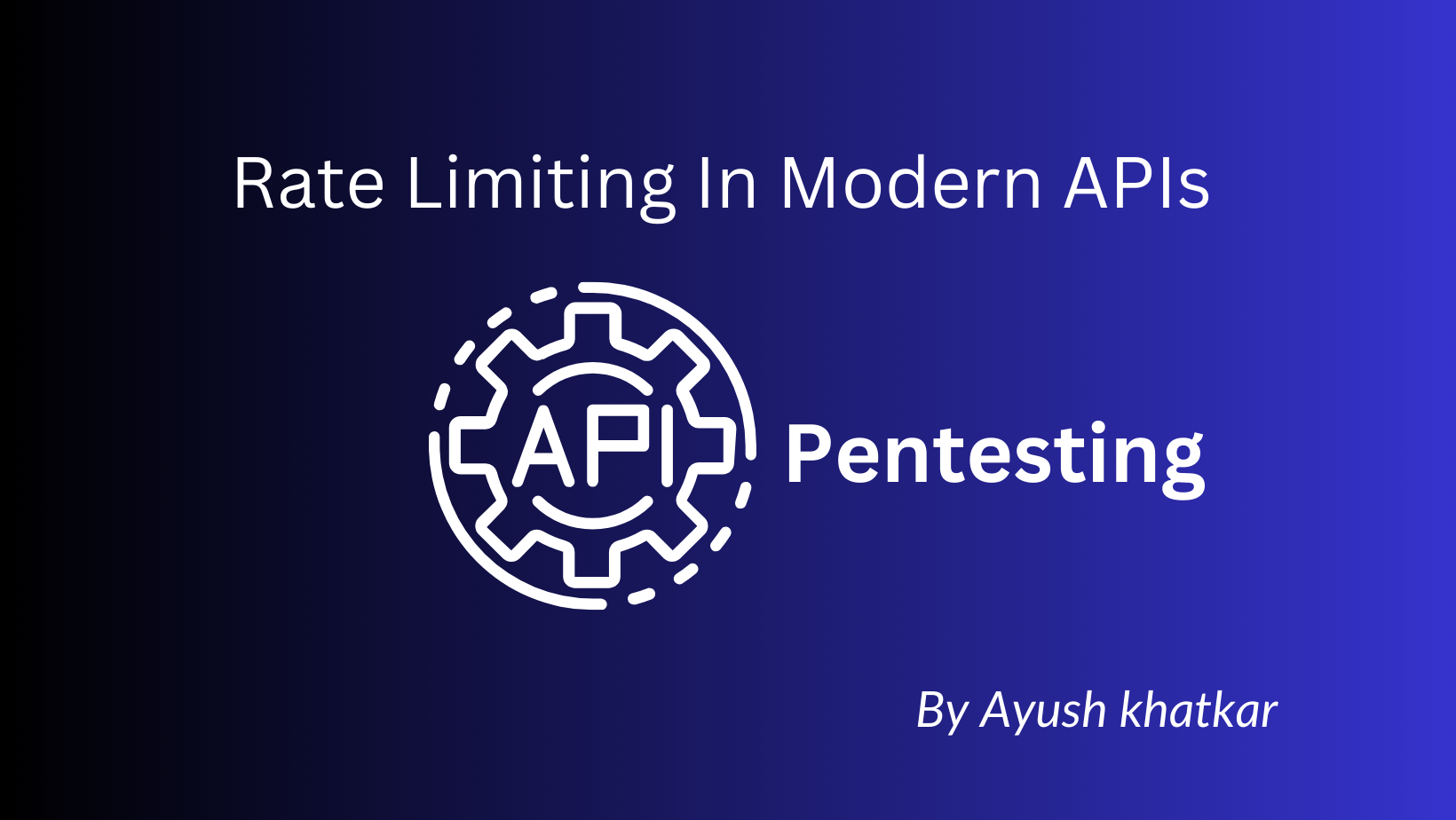
Introduction
Rate limiting is an essential security mechanism in modern APIs. Its purpose is to prevent abuse, protect server resources, and ensure service availability for all users. However, incorrect or weak configurations can lead to vulnerabilities that bug bounty hunters can exploit.
Understanding Rate Limiting
● What is Rate Limiting? A process that limits the number of requests a user or IP can make to an API within a given period.
● Objectives:
○ Prevent denial-of-service (DoS) attacks.
○ Protect against resource abuse.
○ Ensure fair bandwidth distribution.
○ Control infrastructure costs.
● Types of Rate Limiting:
○ User-based: Limits requests per authenticated user.
○ IP-based: Limits requests per IP address.
○ Token-based: Limits requests per API token.
○ Method-based: Limits requests per HTTP method type (GET, POST, etc.).
How Rate Limiting Works
1. Client Identification: The API identifies the client through their IP, API token, user credentials, etc.
2. Request Tracking: The API records the number of requests made by the client within a time period.
3. Limit Enforcement: If the client exceeds the allowed limit, the API rejects additional requests with an HTTP status code 429 (Too Many Requests).
4. Counter Reset: Request counters are reset after a specific time period.
Finding Rate Limiting Issues in APIs
1. Reconnaissance:
○ Analyze Response Headers: Look for headers like X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Reset that indicate the rate limiting configuration.
○ Test Different Endpoints: Verify if rate limiting is applied consistently across all endpoints.
○ Vary Authentication Methods: Check if rate limiting changes when using different authentication methods (API key, token, etc.).
2. Identifying Vulnerabilities:
○ Weak Rate Limiting: If the limit is too high, an attacker could flood the API with requests.
○ Inconsistent Rate Limiting: If rate limiting is applied differently across endpoints or methods, an attacker could find ways to bypass it.
○ Rate Limiting Bypass: Look for ways to bypass rate limiting, such as changing the IP address, using multiple accounts, or manipulating request headers.
Examples of Rate Limiting Vulnerabilities
● Denial-of-Service (DoS) Attack: An attacker can send a large number of requests to exhaust the rate limit and make the API unavailable to other users.
● Restriction Evasion: An attacker can bypass rate limiting restrictions to access data or perform actions that would normally be limited.
● Resource Abuse: An attacker can exploit weak rate limiting to excessively consume server resources, which can affect performance or incur additional costs.
Useful Tools
● Burp Suite: For intercepting and modifying API traffic.
● Postman: For sending requests and analyzing responses.
● Kiterunner: For automating API tests.
Rate limiting is a crucial component of API security. By understanding how it works and how to identify vulnerabilities, bug bounty hunters can contribute to improving web application security and protecting users from potential attacks.
Let's dive into more real-world examples of how to find rate limiting issues in web applications, specifically focusing on APIs:
1. Analyzing Response Headers:
● Scenario: You're testing an API that requires authentication. After a few requests, you notice the response header X-RateLimit-Remaining starts decreasing with each subsequent request.
● Action: Keep making requests until the X-RateLimit-Remaining value reaches zero. The server should then respond with a 429 Too Many Requests status code. This indicates a rate limit has been hit.
● Vulnerability Potential:
○ Weak Rate Limit: If the limit is too high, an attacker could potentially exhaust it and cause a denial-of-service (DoS) condition.
○ Inconsistent Rate Limit: If different endpoints have different rate limits, an attacker could focus on less protected ones.
2. Testing Different Endpoints:
● Scenario: You're interacting with an API that has multiple endpoints (e.g., /users, /orders, /products).
● Action: Send rapid requests to each endpoint and observe if they are all subject to the same rate limiting rules.
● Vulnerability Potential:
○ Inconsistent Rate Limit: If some endpoints have weaker or no rate limiting, they become potential targets for abuse.
3. Varying Authkentication Methods:
● Scenario: An API allows access via both API keys and OAuth tokens.
● Action: Test the rate limiting behavior when using each authentication method.
● Vulnerability Potential:
○ Inconsistent Rate Limit: If the rate limit is different depending on the authentication method, an attacker could exploit the weaker one.
4. Manipulating Request Parameters:
● Scenario: An API uses a parameter like limit to control the number of results returned per request.
● Action: Try manipulating this parameter to see if you can bypass rate limiting by requesting a large number of items at once.
● Vulnerability Potential:
○ Bypass of Rate Limiting: If successful, this indicates a potential way to overload the API.
5. Utilizing Multiple Accounts or IPs:
● Scenario: You suspect an IP-based rate limiting mechanism is in place.
● Action: Make requests from different IP addresses (e.g., using a VPN or multiple devices) or create multiple accounts to see if the rate limit resets.
● Vulnerability Potential:
○ Bypass of Rate Limiting: If successful, this demonstrates how an attacker could evade the restrictions.
6. Fuzzing:
● Scenario: You want to thoroughly test the robustness of the rate limiting implementation.
● Action: Use a fuzzing tool (like Burp Suite Intruder or a custom script) to send a large volume of varied requests with different parameters, headers, and values.
● Vulnerability Potential:
○ Unexpected Behavior: Fuzzing might reveal unexpected behavior in the rate limiting mechanism, such as errors or bypasses.
Additional Tips:
● Look for Clues in Documentation: API documentation might explicitly mention rate limits or give hints about the underlying implementation.
● Monitor Error Messages: Pay close attention to error messages returned by the API, as they might reveal information about rate limiting thresholds or mechanisms.
● Utilize Rate Limiting Testing Tools: Consider tools like Kiterunner, which can automate rate limit testing.
How to make money out of Rate Limiting
We'll examine one such important problem, the rate limiting issue, which seriously jeopardizes password authentication methods. This vulnerability, which is frequently disregarded, can give attackers a simple approach to get around password authentication systems.
Knowing Rate Limiting: The Napping Guard Dog
Rate limitation serves as our defense against brute force attacks, much like a guard dog. However, occasionally even the most capable security dogs doze off, and cybercriminals take advantage of the chance to slip past the guards! Hackers skirt the rate constraints as if they were James Bond, much like in a spy film!
Imagine this: hackers planning their cunning strategy to break the coding, rubbing their hands with delight. Like persistent door-to-door salesman, they release their bots, script kiddies, and other digital miscreants, knocking on the door of password authentication. They yell, "Open up, open up!" and occasionally the door does open, giving them a free pass!
Recognizing the Bug in "Unrestricted Rate Limiting"
Imagine the following situation: you are browsing your online application's Settings and Privacy section when you discover a bug — a weakness in the rate limiting system. This vulnerability can be used by an attacker to change a victim's password and even alter their email address.
How to Reproduce:
1. Navigate to Settings and Privacy -> Accounts: The attacker begins by gaining access to the user's account settings, particularly the "Accounts" section wherever users are able to modify their profile details.
2. Press the Email -> Password link. The attacker then chooses the "Password" option, which allows users to change their account passwords, after selecting the "Email" tab.
3. After entering any random password, select "Next": The attacker clicks "Next" to initiate the password update request after purposefully entering a random wrong password.
4. Catch the Request and Forward it to the Intruder: The attacker uses specialized tools to catch the request before it ever reaches the server. They then reroute this request to "Intruder," a tool, so they can manipulate it even further.
5. Choose the Old Password Position: Using the Intruder tool, the attacker locates the old password parameter's location in the intercepted request, getting ready to launch a brute force assault.
6. Add Password List to Payload: The attacker gathers possible passwords and adds them to Intruder's payload section. We'll employ this list in the brute force attack.
7. Launch the Offensive: The unrelenting portion is about to begin. Because of the weakness, there is no enforcement of the rate limit, so the attacker starts the brute force attack. The hacker begins by attempting each password on the list one at a time until it determines which one is correct.
8. Great! Password Updated: The victim's password is updated with the attacker's choice once the hacker successfully matches a password from the list with the proper one, thereby gaining control of the account.
Consequences: Digital chaos and ATO
A whole new realm of mayhem breaks out as the attacker manages to get beyond the password authentication with unconstrained rate limitation. Now that the victim has changed their password, the attacker has unrestricted access to the account and can make changes without authorization or even manipulate the associated email address. The ramifications are extensive, encompassing identity theft, data breaches, and even damage to the victim's reputation.
A Lucrative Business Emerges:
Let's get started on the thrilling portion now! Equipped with this newfound understanding of the rate limiting defect, my brave bounty hunter embarks on a mission to properly reveal the vulnerability and retrieve the prizes.
First up is Twitter, the massive social media platform. The hunter reports the bug expertly, and to their joy, it is decided that the bug qualifies for a reward! An incredible $700 prize is claimed, which is remarkable for a novice bug bounty participant!
However, the story doesn't finish there! As my confidence as a bounty hunter develops, I explore into additional private projects only to find the same fault hiding in plain sight. I responsibly reveal the vulnerabilities one after another, being paid for my efforts.
As they continue to uncover these low hanging fruit bugs, the treasure chest fills up with $350 from an exclusive private program and $250 from another platform.
The rewards come in steady stream, $100 here, $250 there, and before they realize it, the total exceeds $3,000! Thanks to this seemingly straightforward rate limiting issue, our brave bounty hunter has truly struck gold in the world of bug bounties.
Shutting Down the Rabbit Hole
Let this story encourage aspiring bounty hunters to set out on their own missions, finding valuables by sleuthing down rabbit holes. Together, we can strengthen our digital paradise and make sure that cunning attackers are deterred from attempting low-hanging fruit bugs. Let's celebrate the triumph of expertise and the ethical hacking spirit as we close the gates to chaos!
Happy Hacking
Author: Ayush khatkar is a cybersecurity researcher, technical writer and an enthusiastic pen-tester at Asecurity. Contact here.
#bugbounty #infosec #cybersecurity