Responsive Layouts with CSS Flexbox: Creating Flexible Designs
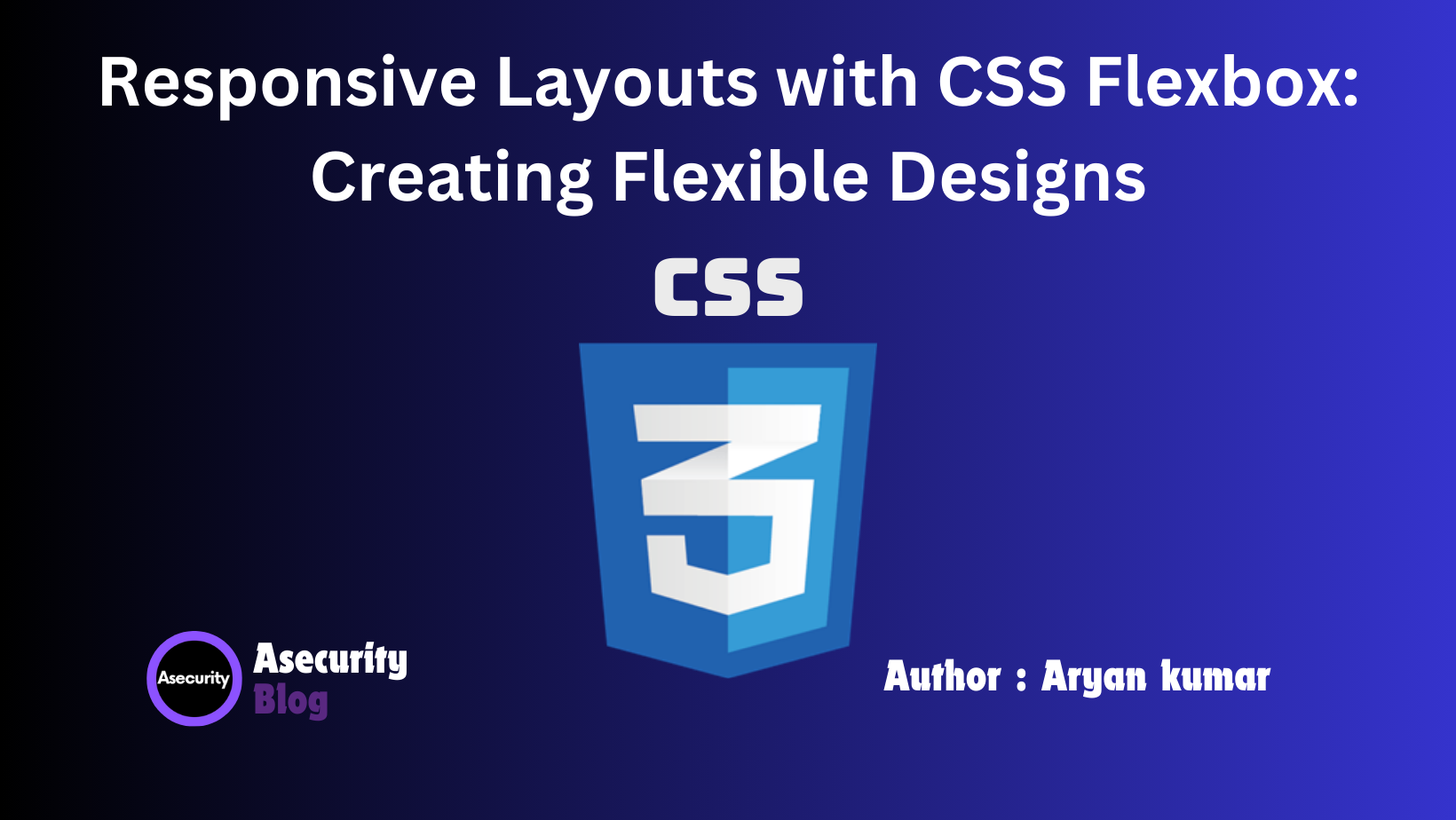
In today's web development landscape, creating responsive layouts is essential for providing a seamless user experience across various devices. CSS Flexbox, short for "Flexible Box Layout," is a powerful layout model that allows developers to create flexible and responsive designs with ease. Whether you're designing for a small mobile screen or a large desktop monitor, Flexbox provides a more intuitive and efficient way to manage the alignment, distribution, and spacing of elements.
Understanding CSS Flexbox
Flexbox is a one-dimensional layout method, meaning it can control the layout in one direction—either as a row or a column. This characteristic makes Flexbox an excellent choice for building complex layouts without having to deal with float or positioning tricks. Flexbox was designed to manage space distribution and alignment of items within a container, allowing for more control over how elements resize and reflow as the viewport changes.
Key Concepts:
- Flex Container and Flex Items: The Flexbox layout is based on two main components:
- Flex Container: The parent element that houses the flex items. It's defined by applying
display: flex;
to an element. - Flex Items: The direct children of a flex container. These items are the elements you want to arrange within the container.
- Flex Container: The parent element that houses the flex items. It's defined by applying
- Main Axis and Cross Axis:
- The main axis is the primary axis along which flex items are placed. It can be horizontal or vertical, depending on the
flex-direction
property. - The cross axis is perpendicular to the main axis. The alignment of flex items on the cross axis is controlled by properties like
align-items
andalign-content
.
- The main axis is the primary axis along which flex items are placed. It can be horizontal or vertical, depending on the
- Flex Properties:
flex-direction
: Determines the direction of the main axis (row
,row-reverse
,column
,column-reverse
).justify-content
: Aligns items along the main axis (flex-start
,flex-end
,center
,space-between
,space-around
,space-evenly
).align-items
: Aligns items along the cross axis (flex-start
,flex-end
,center
,baseline
,stretch
).flex-wrap
: Allows flex items to wrap onto multiple lines (nowrap
,wrap
,wrap-reverse
).align-content
: Aligns flex lines within the flex container when there is extra space (flex-start
,flex-end
,center
,space-between
,space-around
,stretch
).
Creating a Simple Flexbox Layout
Let’s start with a basic example to understand how Flexbox works. Imagine we want to create a simple navigation bar with evenly spaced items:
<div class="navbar">
<div class="nav-item">Home</div>
<div class="nav-item">About</div>
<div class="nav-item">Services</div>
<div class="nav-item">Contact</div>
</div>
To style this with Flexbox, you would use the following CSS:
.navbar {
display: flex;
justify-content: space-between;
background-color: #333;
padding: 10px;
}
.nav-item {
color: white;
padding: 10px;
text-decoration: none;
}
In this example:
display: flex;
makes the.navbar
a flex container.justify-content: space-between;
ensures the items are spaced evenly within the container.- Additional styling enhances the visual appearance of the navigation bar.
Building a Responsive Layout
Flexbox truly shines when creating responsive designs. Let's modify the above navigation bar to make it responsive:
.navbar {
display: flex;
flex-direction: column;
align-items: center;
background-color: #333;
padding: 10px;
}
@media (min-width: 768px) {
.navbar {
flex-direction: row;
justify-content: space-between;
}
}
Here, we’ve:
- Set the default
flex-direction
tocolumn
for mobile devices, stacking the items vertically. - Used a media query to switch the
flex-direction
torow
on larger screens (768px and up), making it a horizontal navigation bar.
Advanced Flexbox Techniques
Flexbox offers numerous advanced techniques for creating even more sophisticated layouts:
- Nested Flex Containers: Flex containers can be nested within each other to create intricate layouts. For example, a main container could use Flexbox to arrange columns, and each column could also be a flex container for arranging rows within it.
- Alignment and Distribution: By mixing and matching properties like
align-self
,order
, and usingauto margins
, you can achieve complex alignments and distributions, such as centering items both horizontally and vertically with ease. - Responsive Adjustments: Flexbox, combined with CSS media queries, allows for dynamic adjustments based on screen size. This combination is a powerful tool for ensuring layouts remain functional and aesthetically pleasing on all devices.
Conclusion
CSS Flexbox is an invaluable tool for modern web development. It simplifies the process of creating responsive layouts, making it easier to build designs that adapt seamlessly to various screen sizes. Whether you’re just starting with web design or looking to enhance your skills, mastering Flexbox is a critical step in creating flexible, user-friendly websites. Experiment with different properties, combine them creatively, and watch as your layouts transform from static to dynamic, enhancing the overall user experience.
Next up in this series, we’ll delve into Advanced CSS Grid Techniques, exploring the Grid layout system for designing even more complex web page layouts. Stay tuned!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript