Working with Forms: Validating and Processing User Input in JavaScript
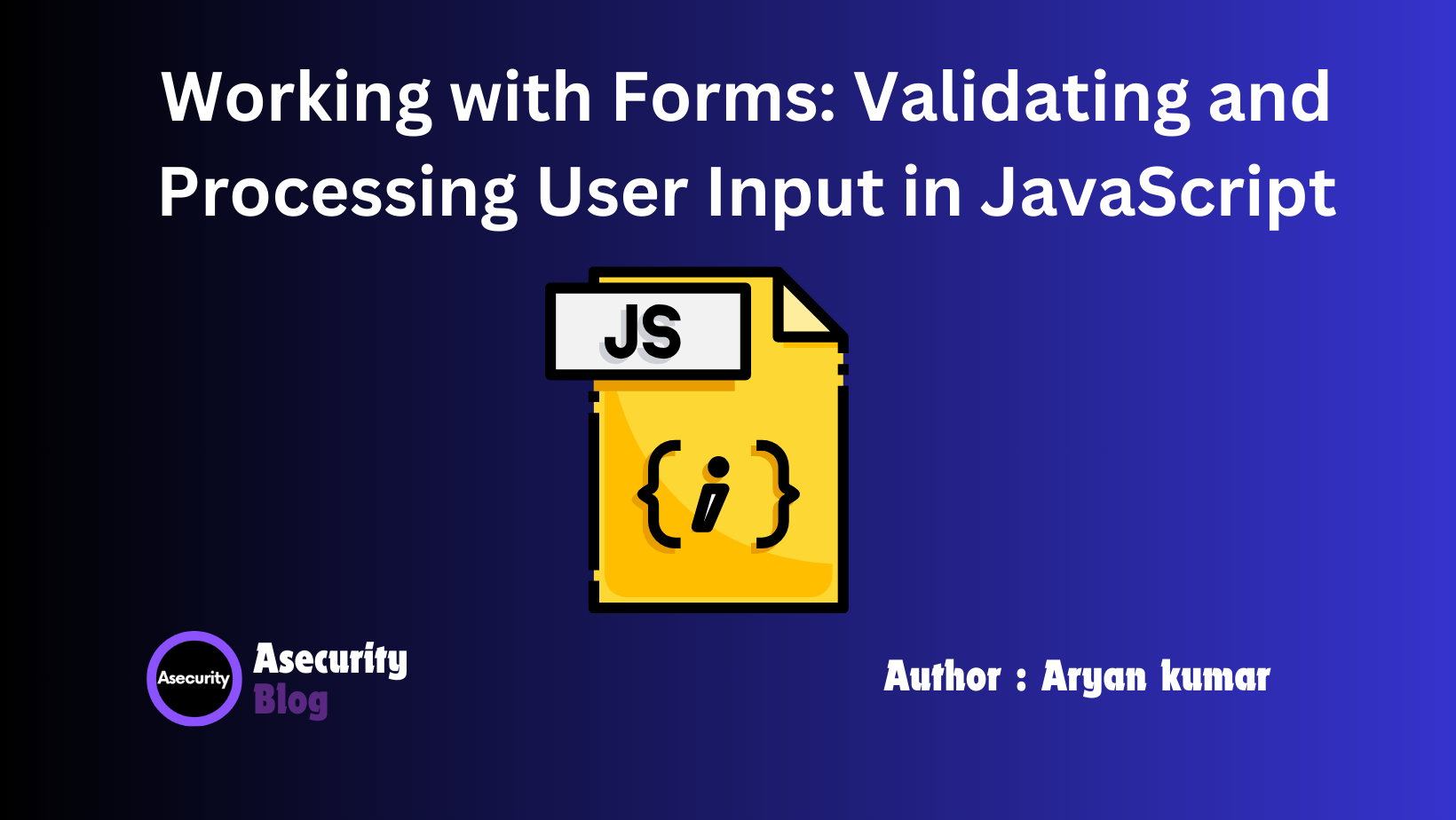
Forms are a fundamental part of web development, enabling users to interact with web pages by providing input data. Whether it’s signing up for a newsletter, logging into an account, or filling out a survey, forms are everywhere on the web. In this blog, we’ll explore how to work with forms in JavaScript, focusing on validating user input and processing it effectively. This guide is beginner-friendly, so let’s dive in!
Understanding Forms in Web Development
Forms are used to collect data from users, which can then be sent to a server for processing. However, before submitting the data, it's crucial to validate it to ensure it’s correct and formatted properly. JavaScript provides powerful tools for validating and processing forms directly on the client side, improving user experience by providing instant feedback.
Basic Structure of a Form
Here’s a simple HTML form that we’ll use throughout this blog:
<form id="registrationForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Register</button>
</form>
This form contains:
- A text input for the username.
- An email input for the email address.
- A password input for the password.
- A submit button to send the form data.
Adding Event Listeners to Handle Form Submission
To work with forms in JavaScript, we need to add event listeners that can detect when the form is submitted. This allows us to intercept the submission, validate the input, and either proceed or stop the process based on the validation results.
// Select the form element
const form = document.getElementById('registrationForm');
// Add a submit event listener to the form
form.addEventListener('submit', function(event) {
// Prevent the default form submission behavior
event.preventDefault();
// Validate the form inputs
validateForm();
});
Validating Form Input with JavaScript
Validation is a crucial step to ensure the data collected is useful and formatted correctly. Let’s look at some common validation techniques:
- Checking if Fields Are Empty: Ensure that required fields are filled out.
- Validating Email Format: Use regular expressions to check if the email is in the correct format.
- Password Strength: Check if the password meets the required length and contains numbers, letters, and special characters.
Example: Simple Form Validation
// Function to validate form inputs
function validateForm() {
const username = document.getElementById('username').value;
const email = document.getElementById('email').value;
const password = document.getElementById('password').value;
// Check if the username is empty
if (username === '') {
alert('Username cannot be empty!');
return;
}
// Validate the email format using a regular expression
const emailPattern = /^[^ ]+@[^ ]+\.[a-z]{2,6}$/;
if (!emailPattern.test(email)) {
alert('Please enter a valid email address!');
return;
}
// Check password strength
if (password.length < 8) {
alert('Password must be at least 8 characters long!');
return;
}
alert('Form submitted successfully!');
}
In this example:
- We first check if the username field is empty and alert the user if it is.
- We validate the email using a regular expression to ensure it follows a typical email format.
- We check the password to ensure it’s at least 8 characters long.
Preventing Default Form Behavior
JavaScript’s event.preventDefault()
method is essential in form handling. By default, when a form is submitted, the page refreshes, which can be frustrating for users, especially if errors occur. Using preventDefault()
stops the form from automatically submitting, allowing you to validate the inputs first.
Processing User Input
Once the form data is validated, you can process it, such as sending it to a server via an API. Here’s a basic example using fetch()
to send form data to a server:
// Function to process form data
function processFormData() {
const formData = new FormData(form);
// Example of sending form data to a server
fetch('https://example.com/submit', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => {
console.log('Success:', data);
})
.catch(error => {
console.error('Error:', error);
});
}
In this example:
- We use the
FormData
object to collect data from the form inputs. - We send the data to a server endpoint using the
fetch()
API with a POST request. - The server response is logged to the console for success or error handling.
Best Practices for Working with Forms
- Client-Side and Server-Side Validation: Always validate data on both the client side (JavaScript) and the server side for security and reliability.
- Provide User Feedback: Use alerts or visual indicators to guide users on what needs to be corrected.
- Keep Forms Simple: Avoid cluttering forms with too many fields. Keep them simple and user-friendly.
Conclusion
Mastering form validation and processing with JavaScript is a fundamental skill in web development. By understanding how to handle form events, validate inputs, and send data to the server, you can create robust, interactive forms that enhance the user experience. Keep practicing, experiment with different validation techniques, and soon you’ll be comfortable handling any form challenge in JavaScript!
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript