Writing Reusable Code: Mastering JavaScript Functions
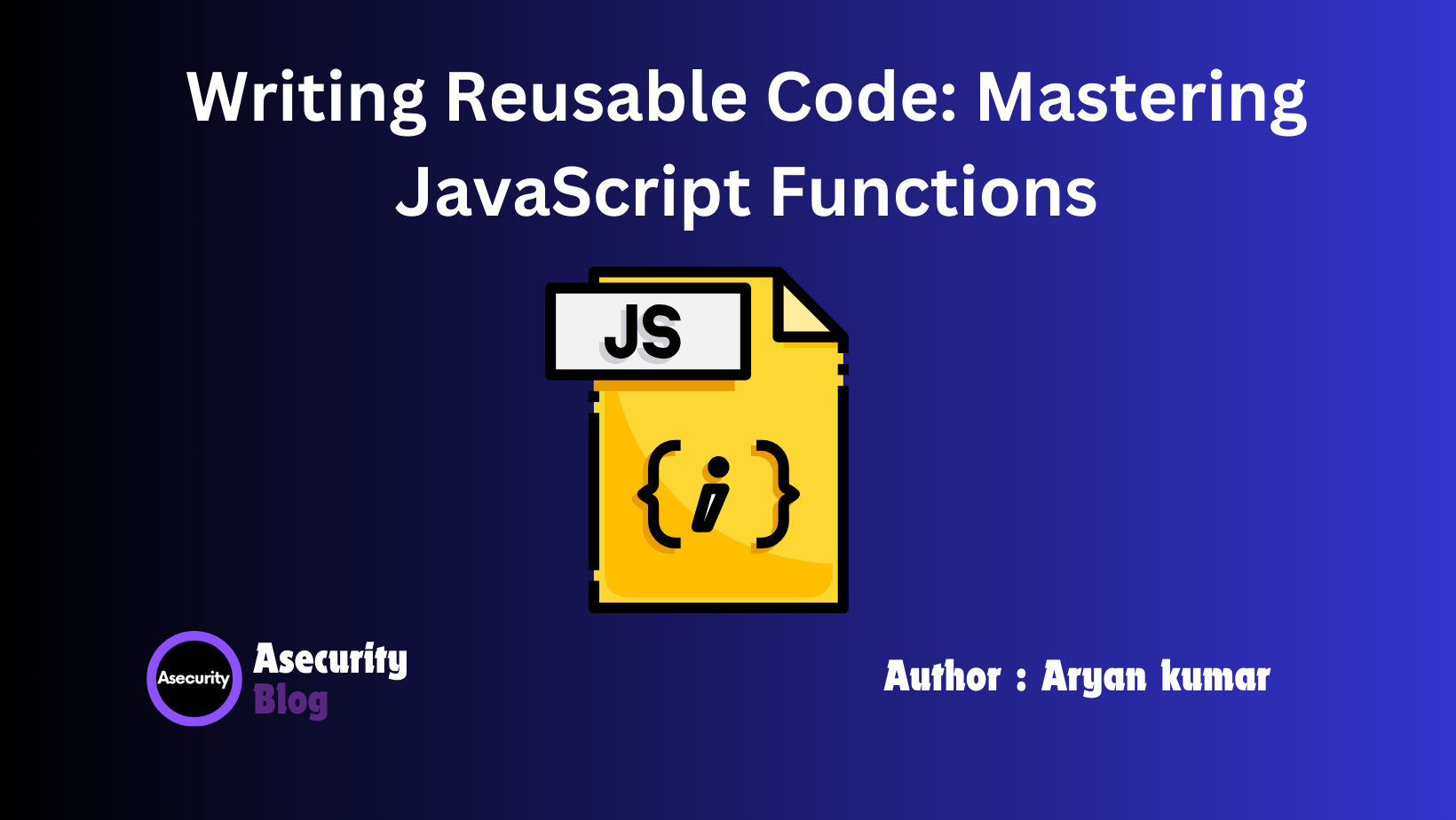
As you dive deeper into JavaScript, you’ll encounter situations where the same block of code is used repeatedly. Copying and pasting the same code over and over again is not only tedious but also makes your code less efficient and harder to maintain. This is where functions come into play. Functions allow you to write reusable code, making your programs cleaner, more efficient, and easier to manage.
In this blog, we’ll explore JavaScript functions in depth, helping you master one of the most essential tools in your programming toolkit.
What Are Functions in JavaScript?
A function is a reusable block of code designed to perform a specific task. Instead of writing the same code multiple times, you can define a function once and use it wherever needed.
Here’s the basic syntax for declaring a function in JavaScript:
function functionName() {
// Code to be executed
}
Let’s look at a simple example of a function that prints a message to the console:
function greet() {
console.log("Hello, world!");
}
In the example above, the function greet()
contains one line of code that logs a message. To use this function, you would simply call it by its name:
greet(); // Outputs: Hello, world!
By defining the function once, you can now call greet()
anytime you need to print that message without rewriting the code.
Function Parameters and Arguments
Functions become even more powerful when they can accept parameters. Parameters allow you to pass information into functions, making them more flexible and adaptable.
Here’s an example of a function with parameters:
function greet(name) {
console.log("Hello, " + name + "!");
}
In this case, name
is a parameter. You can pass different values to the function when you call it, which are known as arguments:
greet("Alice"); // Outputs: Hello, Alice!
greet("Bob"); // Outputs: Hello, Bob!
With parameters, you can customize the behavior of a function based on the input it receives.
Function Return Values
In addition to performing tasks, functions can also return values. This means that after executing the code inside the function, a result is sent back to the part of the program that called the function.
Here’s how you can return a value from a function:
function add(a, b) {
return a + b;
}
This function takes two numbers as parameters, adds them together, and returns the result. You can store the returned value in a variable or use it directly:
let sum = add(5, 3);
console.log(sum); // Outputs: 8
By using return values, functions can perform calculations and provide results that other parts of your program can use.
Function Expressions and Arrow Functions
In JavaScript, functions are first-class citizens, meaning you can assign them to variables, pass them as arguments, and return them from other functions. One way to define a function is through a function expression:
let multiply = function(a, b) {
return a * b;
};
You can call this function by referencing the variable name:
console.log(multiply(4, 5)); // Outputs: 20
With the introduction of ES6, JavaScript also introduced arrow functions, a more concise way to write functions:
let multiply = (a, b) => a * b;
Arrow functions work similarly to regular functions but have some differences, particularly in how they handle the this
keyword. They are particularly useful for short, simple functions.
Anonymous Functions and Callback Functions
Sometimes, you’ll need a function that doesn’t have a name. These are called anonymous functions. They are often used as callback functions, which are functions passed into another function as an argument and executed later.
Here’s an example of an anonymous function used as a callback in the setTimeout()
function:
setTimeout(function() {
console.log("This message appears after 2 seconds.");
}, 2000);
In the example above, the anonymous function is executed after 2 seconds. Callbacks are essential in JavaScript, especially when dealing with asynchronous operations like fetching data from a server.
Higher-Order Functions
JavaScript functions can be higher-order functions, which means they can take other functions as arguments or return them as values. Higher-order functions are a powerful feature of JavaScript that allow for more abstract and reusable code.
For example, JavaScript array methods like map()
, filter()
, and reduce()
are higher-order functions:
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(function(number) {
return number * 2;
});
console.log(doubled); // Outputs: [2, 4, 6, 8, 10]
In this example, map()
takes a function as an argument and applies it to each element in the array, returning a new array with the results.
Conclusion
Mastering functions is a critical step toward writing efficient, reusable, and clean code in JavaScript. By understanding how to create and use functions, work with parameters, return values, and leverage higher-order functions, you’ll be able to write more powerful and flexible programs.
In our next blog, we’ll dive into Control Structures in JavaScript, where you’ll learn how to make decisions in your code with conditionals and loops. Stay tuned!
This blog introduces the concept of functions and builds upon each feature step by step to provide a complete understanding of how JavaScript functions operate.
Happy coding!
Author: Aryan Kumar is a web developer specializing in HTML, CSS, and JavaScript, working at Asecurity. Contact here (Instagram) : @aryan_geek .
#webdevelopment #html #css #javascript